Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial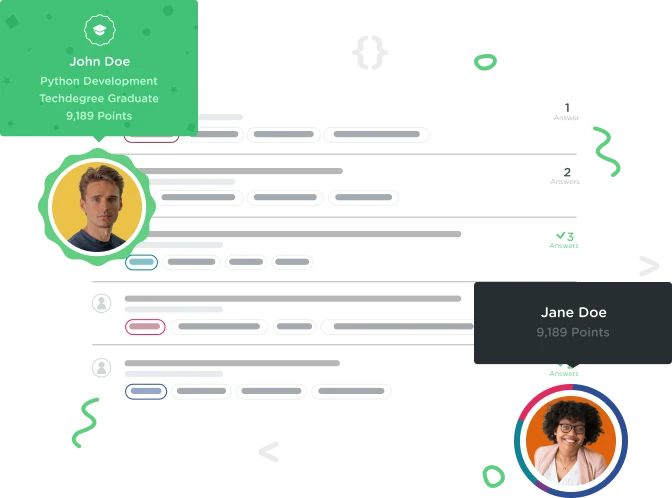

John Weland
42,478 PointsHow to properly account for errors in jQuery's .ajax() call
I've noticed a little craziness in trying to properly check for errors in my ajax calls to Google Maps API.
Google Maps API returns the following status codes "OK", "ZERO_RESULTS", "OVER_QUERY_LIMIT", "REQUEST_DENIED", "INVALID_REQUEST", and "UNKNOWN_ERROR".
So in theory I should make my call like this...
zipcode = 78130;
function geocoder (zipcode) {
var url = "http://maps.googleapis.com/maps/api/geocode/json?address=" + zipcode + "&sensor=false";
$.ajax(url, {
type : "GET",
success : function (response) {
console.log("yay!");
},
error : function () {
if (response.status === "ZERO_RESULTS") {
console.log("No results found");
} else if (response.status === "OVER_QUERY_LIMIT") {
console.log("We are over the query limit");
} else if (response.status === "REQUEST_DENIED") {
console.log("Request, denied");
} else if (response.status === "INVALID_REQUEST") {
console.log("address, components or latlng maybe missing");
} else if (response.status === "UNKNOWN_ERROR") {
console.log("Something went wrong, terribly terribly wrong.");
}
}
});
}
Yet the only way I can seem to make my error code work properly is to move them all into the success : portion of the call like so.
zipcode = 78130;
function geocoder (zipcode) {
var url = "http://maps.googleapis.com/maps/api/geocode/json?address=" + zipcode + "&sensor=false";
$.ajax(url, {
type : "GET",
success : function (response) {
if(response.status === "OK"){
console.log("yay");
} else if (response.status === "ZERO_RESULTS") {
console.log("No results found");
} else if (response.status === "OVER_QUERY_LIMIT") {
console.log("We are over the query limit");
} else if (response.status === "REQUEST_DENIED") {
console.log("Request, denied");
} else if (response.status === "INVALID_REQUEST") {
console.log("address, components or latlng maybe missing");
} else if (response.status === "UNKNOWN_ERROR") {
console.log("Something went wrong, terribly terribly wrong.");
}
error : function () {
// What is the point of the error portion of the ajax call?
}
});
}
With the code like this, what is the point of the error: portion of the ajax call because even the status codes are received in the success portion. For testing errors I simply added random characters to the zipcode so it wasn't valid. With the code calling all statuses in the success portion of the ajax call I accurately return errors.
I am stumped, What is the error portion of the call good for?
1 Answer
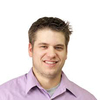
Kevin Korte
28,148 PointsI'm going to take a shot at this one, but I believe you are confusing a failed ajax request, and a failed google maps attempt.
When you make an ajax call, and you get a response back from google, wouldn't that still be a successful callback? You asked google for information, google received it, processed it, and sent something back....that's a success! Even if what you get back is an error from google, the call itself was successful.
The error portion of the ajax call would be for situations where you never even were able to hit google. Maybe the URL param was wrong, maybe googles API service is down, maybe it times out for whatever reason. This would be a failed ajax call since you have no response.
John Weland
42,478 PointsJohn Weland
42,478 PointsI was heading that direction in my thought process but couldn't think of an example of a proper
the broken link scenario works (I just tried it) so now I need to figure out how best to check/output errors.
Kevin Korte
28,148 PointsKevin Korte
28,148 PointsSounds good. Ajax can be tricky trying to cover your bases. I think the more simple and graceful you can error out, the better. Easier said than done I know. Good luck!
I don't envy your problem..lol