Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial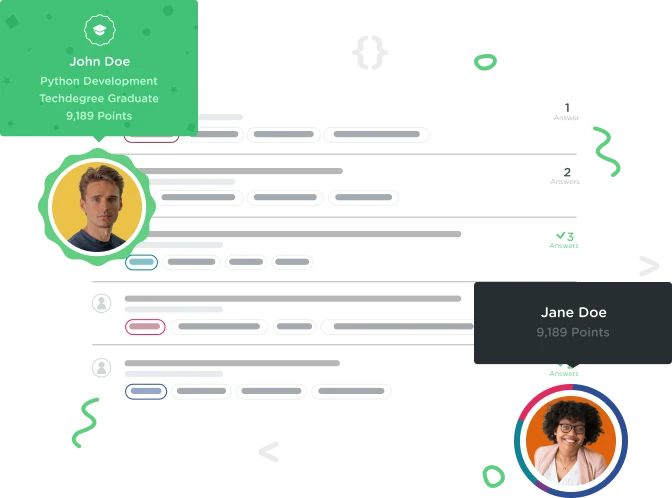

Brian DeShazer
4,527 Pointshow to put an object into an array
not sure why this isn't working
var objects = [
{shapes: {triangles: 2, squares: 3}},
{dogs: {mutts: 2, puppies: 3}},
{jobs: {time: 3, cop: 1}}
];
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Damien Watson
27,419 PointsHi Brian,
Its asking for 3 objects in the array with 2 items each. What you have done will work, but not what was asked. Check the below:
var objects = [
{triangles: 2, squares: 3},
{mutts: 2, puppies: 3},
{time: 3, cop: 1}
];

Mike West
9,163 PointsHello Brian-
To get the code to work you need to remove the "shapes", "dogs" and"jobs" from each object. The array is the only element that needs a name declaration e.g. "var objects". All you need to do in each object is place two key and property pairs. If you were to use strings as your property values you would need to make sure that they are in single or double quotes. Hope this helps Your code should look like this:
var objects = [
{triangles: 2, squares: 3},
{mutts: 2, puppies: 3},
{time: 3, cop: 1}
];

Matt F.
9,518 PointsHi Brian,
The array of objects that you currently have in your post is valid JavaScript.
I think it really improves readability and reasonability when you add whitespace through line breaks and indentations, like this:
var objects = [
{
shapes:
{
triangles: 2,
squares: 3
}
},
{
dogs:
{
mutts: 2,
puppies: 3
}
},
{
jobs:
{
time: 3,
cop: 1
}
}
];
When dealing with arrays of objects, however, you may be adding another layer than expected.
For example, to access the value of triangles, you would need to first target index 0:
objects[0[
then target shapes:
objects[0]['shapes']
then finally target triangles property within the shapes object:
objects[0]['shapes']['triangles']
Similarly to access the value of time, you would write:
objects[2]['jobs']['time']