Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial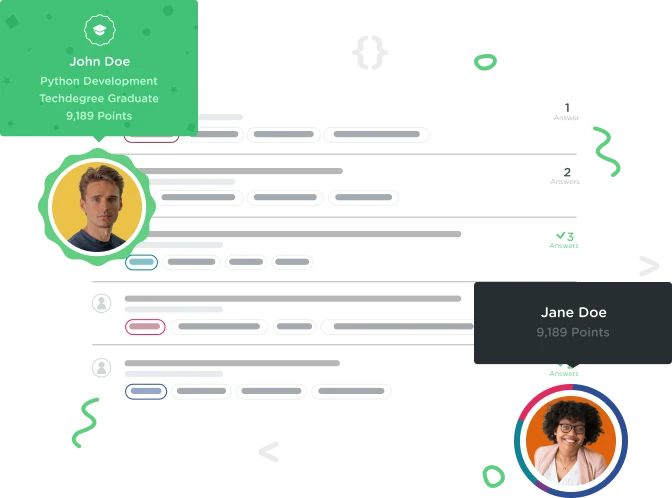

Mohamed Mohamoud
1,015 Pointshow to put numbers as argument in function?
can i put a number as an argument in a function? how?
please explain if you can?
thanks.
def even_odd(7):
return(False)
2 Answers

Luke Armstrong
5,932 PointsNo, you cannot use numbers as arguments in a function.
When you define a function, the arguments are essentially empty placeholders (variables) that you use to tell your function what to do. Then, when you call the function, you give it the information to fill those variables.
Example:
# first I define the function:
def even_odd(x): #x can be called anything you want, so long as it follows the rules of variable names
if x % 2: # this will happen if the number is odd
print("{} is odd!".format(x))
else: # this happens if the number is not odd
print("{} is even!".format(x))
# here we call the function
even_odd(3)
The above part lets you use whatever numbers you want every time you call the function. In this case I used 3, but you can replace that with any number you like.
So again, you cannot use numbers as the argument name (since it is a variable) but you can pass numbers into a function by using an argument. Make sense?
Hope this helps!

Luke Armstrong
5,932 PointsI'll give you a breakdown of it:
if x % 2: # this is the same as saying if x % 2 is True, but shorter.
# x % 2 will divide x by 2 and tell me the remainder
# so 5 divided by 2 is 2 with a remainder of 1, so the formula gives me 1 (which is truthy)
# any odd number will give 1, and any even number will give 0
# since "if x % 2:" is just asking if something is truthy, it will work for odd numbers
print("{} is odd!".format(x)) #the .format(x) replaces the {} with x
# there are lots of uses for this, and you'll get to it soon
else: # this block happens if the x % 2 does not come back "truthy".
# basically, if the number is odd, the 'if' happens, otherwise the 'else' happens.
print("{} is even!".format(x)) # same as last time, the {} is replaced with x
So you can use the {} and .format() for any variable. It's very useful when you can take input from a user and want to display it, or part of it. Don't worry about it too much though, as you'll learn about it soon if you keep on the python track!
Mohamed Mohamoud
1,015 PointsMohamed Mohamoud
1,015 Pointsyh that makes sense thanks very much,
also if i want to solve this question, how would i go about doing it?
Mohamed Mohamoud
1,015 PointsMohamed Mohamoud
1,015 Pointsalso where you said: if x % 2: # this will happen if the number is odd print("{} is odd!".format(x)) wouldnt this print {} for every number that you divide by 2? how would it know whether its odd or even??
is it to do with the number being an integer or a float??
thanks again.