Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial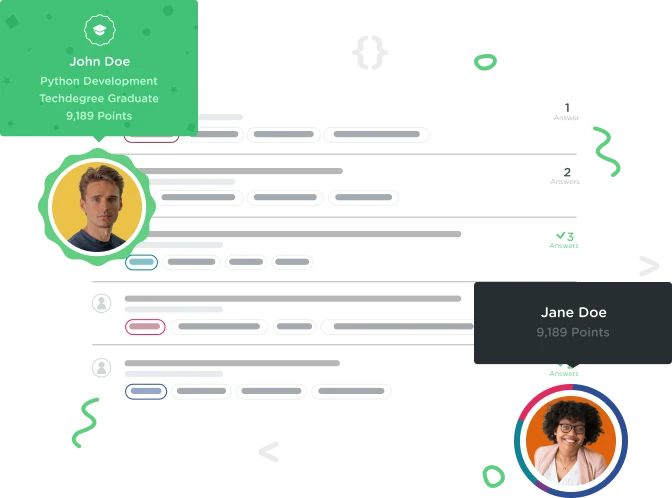

Nader Alharbi
2,253 PointsHow to remove items regardless of upper or lower cases?
Hello,
I am having trouble in removing the item because the remove function is case sensitive. Is there a way to do it?
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pickup at the store?")
print("""
Enter 'SHOW' to show the list.
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'REMOVE' to remove an item from the list
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should i add {}?\n"
"Press Enter to add at the end of the list\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
if position == False:
raise ValueError
except ValueError:
position = None
if position is not None:
shopping_list.insert(position - 1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here is your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
def remove_from_list():
show_list()
what_to_remove = input("What would you like to remove?\n ")
try:
shopping_list.remove(what_to_remove)
except ValueError:
pass
show_list()
show_help()
while True:
new_item = input("> ")
if new_item.upper() == "DONE" or new_item.upper() == "QUIT":
break
elif new_item.upper() == "HELP":
show_help()
continue
elif new_item.upper() == "SHOW":
show_list()
continue
elif new_item.upper() == "REMOVE":
remove_from_list()
continue
add_to_list(new_item)
Thank you.
2 Answers
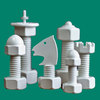
Steven Parker
231,269 PointsYou're already doing case-insensitive comparisons for the command words like "SHOW" by converting the case of the search item. You can use the same technique when comparing two variables by converting them both in the comparison.
This might require using a loop to find the item, and then using "delete" to remove the item by index.

gabrielle moran
1,985 PointsYou can deal with the user entering the item to be removed in uppercase by making your what_to_remove = input("Blah blah blah").lower() Not sure if that was what you were asking, but I have just done that with my code

Nader Alharbi
2,253 PointsThis could work if you force all the items in the list to be upper or lower cases which i don't feel that the user would appreciate it.
But if i had this option only, then i would use capitalize() instead of lower or upper which will make the first letter upper and the rest lower.
Nader Alharbi
2,253 PointsNader Alharbi
2,253 PointsThe command "Remove" is case-insensitive. What i want is to remove banana by typing "BaNAnA".
Can i make shopping_list.remove(what_to_remove) case-insensitive. I also don't want to convert the users input to lower or upper cases inside the list. If the user want to type ApPle then he shall see ApPle in his list.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe "remove" method is case-sensitive. So it won't do what you want by itself.
I wasn't suggesting you change the case of the input before storing it, but changing the case before comparing it to the stored values (which you would also change so they can match).
Then if you determine the index of a match, you can use delete to remove the item at that index.
Nader Alharbi
2,253 PointsNader Alharbi
2,253 PointsBrilliant Sir!
i was testing for 2 hours and i took a break, when i came back i just deleted one line and it works like a charm. My function will remove all cases of any item from the list now :)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsGood job!
And glad I could help.
Happy coding!