Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial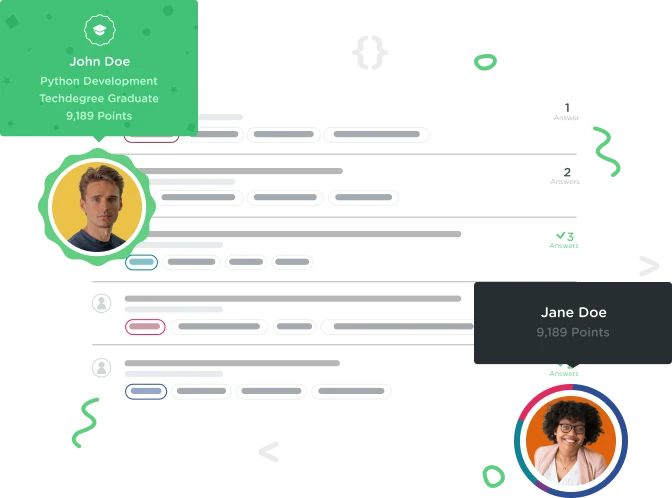

Joan Guerra Makaren
4,067 PointsHow to remove left margin or padding?
How do you remove the left white space in images so all fits perfect with no spaces in the screen?
I mean this: http://puu.sh/aJ22I/2ab5c5e2c9.png
3 Answers

Stone Preston
42,016 PointsOk I just did this fairly easily with little code and some storyboard work.
EDIT: dont name the imageView property imageView, name it something different like myImageView. naming it imageView causes problems
First remove the current prototype cell by selecting it in your storyboard and then pressing delete.
After you delete it, search for cell in the object library and then drag it on to your tableView near the top.
Then drag an image view on to that cell and size it so that its as tall as the cell and square. then move it over so that its right on the edge.
add a title label and size it so that it extends all the way to the right side of the table view then under that add a subtitle label that has a smaller font size and a grey color and also extends to the far side of the tableView.
you can set the cell identifier to whatever you want in the attributes inspect, I named mine "customCell"
then create a new class called THCustomCell and make it a subclass of UITableViewCell. After creating it set the class of your prototype cell to be THCustomCell using the identity inspector.
after setting the class of your cell open the assistant editor and control click and drag from the image view to the header file of your new class, call the outlet myImageView (if you call it imageView it messes things up since the superclass has a property named imageView as well so name it something else)
then control click and drag from the title label to the header, call it titleLabel
then control click and drag from the subtitleLabel to the header, call it subtitleLabel
this is what my header looked like after connecting the outlet properties:
#import <UIKit/UIKit.h>
@interface THCustomCell : UITableViewCell
@property (weak, nonatomic) IBOutlet UILabel *titleLabel;
@property (weak, nonatomic) IBOutlet UILabel *subtitleLabel;
@property (weak, nonatomic) IBOutlet UIImageView *myImageView;
@end
after that switch to your viewController file. import your new custom cell class
#import "THCustomCell.h"
then in cellForRowAtIndexPath you need to set the cell identifier to whatever you named it and instantiate the cell using your new class
//in cellForRowAtIndexPath
//use the identifier you gave it in your storyboard
static NSString *CellIdentifier = @"customCell";
//use your new custom cell class
THCustomCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
then configure the cell
// Configure the cell...
BlogPost *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
//set the imageView property of your custom cell
if ( [blogPost.thumbnail isKindOfClass:[NSString class]]) {
NSData *imageData = [NSData dataWithContentsOfURL:blogPost.thumbnailURL];
UIImage *image = [UIImage imageWithData:imageData];
cell.myImageView.image = image;
} else {
cell.myImageView.image = [UIImage imageNamed:@"treehouse"];
}
//set the title label of your custom cell
cell.titleLabel.text = blogPost.title;
//set the subtitleLabel of your custom cell
cell.subtitleLabel.text = [NSString stringWithFormat:@"%@ - %@", blogPost.author, [blogPost formattedDate]];
return cell;
below is my full implementation of the cellForRowAtIndex path method:
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"customCell";
SPCustomCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
// Configure the cell...
BlogPost *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
if ( [blogPost.thumbnail isKindOfClass:[NSString class]]) {
NSData *imageData = [NSData dataWithContentsOfURL:blogPost.thumbnailURL];
UIImage *image = [UIImage imageWithData:imageData];
cell.myImageView.image = image;
} else {
cell.myImageView.image = [UIImage imageNamed:@"treehouse"];
}
cell.titleLabel.text = blogPost.title;
cell.subtitleLabel.text = [NSString stringWithFormat:@"%@ - %@", blogPost.author, [blogPost formattedDate]];
return cell;
}
you also may have to create your segue from the cell to the web view if it got deleted when you removed the old prototype.
a lot of steps but not too bad

Joan Guerra Makaren
4,067 PointsExacly... so still waiting proper answer

Stone Preston
42,016 Pointschanging the layout of the default UITableViewCell is difficult. Its probably easier to delete the current prototype cell on your tableView in storyboard, drag a new one on, design a custom UITableViewCell prototype in your storyboard with an imageView positioned right next to the edge, a title label, and then a subtitle label underneath that, create a custom class, hook those outlets up to your custom class, give your cell an identifeir in storyboard, then instantiate a cell using your identifier in cell for row at index path
Eric M
2,242 PointsEric M
2,242 PointsThis is a really thorough answer. Thanks for taking the time!