Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial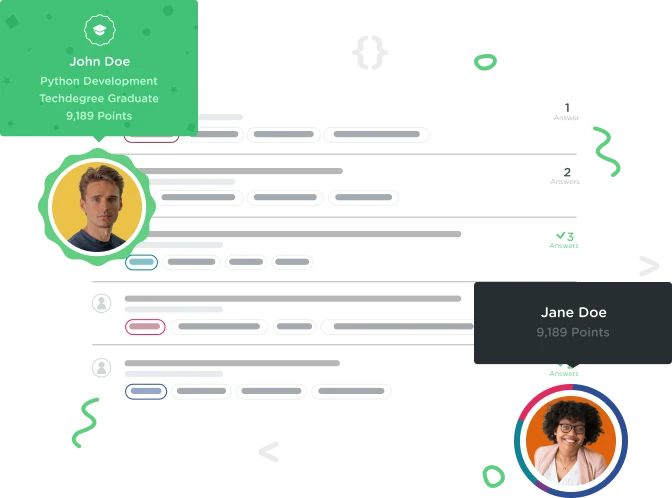

Jason Peay
16,515 PointsHow to Repeat a Loop?
In this challenge, you will create a while loop that prints to the document 26 times. We've added a variable named count, use it to track the number of times the loop runs. Don't forget to use the document.write() method inside the loop.
var count = 0;
while ( counter < 26 ) {
var count = 26;
document.write(count);
counter ++;
}
2 Answers
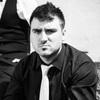
Billy Bellchambers
21,689 PointsYou are pretty close however there is an issue with your code.
Your code and issues.
var count = 0;
while ( counter < 26 ) {
var count = 26; //this line set count to 26 on first entry into while loop this means the only value of count that writes to document is 26, after this first pass count = 27 the while argument no longer passes and no further loops are made.
document.write(count);
counter ++; //you need to increment the var count (counter isnt currently defined or even part of the argument tested in the while loop)
}
Correct code as follows
var count = 0;
while (count<26) {
document.write(count);
count++;
}

Jason Peay
16,515 PointsThanks for your help, Billy
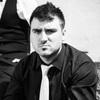
Billy Bellchambers
21,689 PointsYour welcome.
Happy coding

Charles Smith
7,575 PointsLet's look at the code:
var count = 0; // sets a count variable
while ( counter < 26 ) { // sets our condition against a --counter-- variable, which we haven't defined yet
var count = 26; // sets the value of count to 26
document.write(count); // writes the value of count, which should be 26, to the page
counter ++; // increments the undefined counter variable.
}
Instead, let's try:
var counter = 0; // the variable we'll use to count how many times the loop runs and print to the page
while ( counter < 26 ) { // this line sets the condition which will break the loop
document.write(counter); // writes the current value of counter to the page
counter ++; // increments the counter by 1
}
Hope that helps!
George Kiknadze
837 PointsGeorge Kiknadze
837 PointsIn your code there is a mistake:
I these is the right answer :)
I hope I helped you.