Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial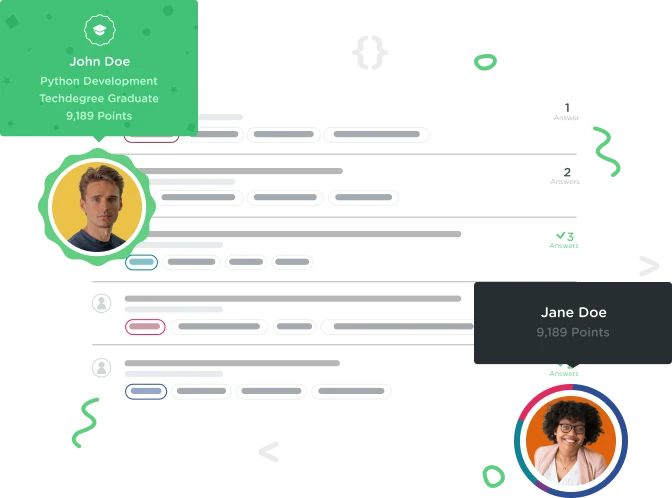
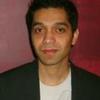
Mohammed Hossen
5,591 PointsHow to resolve assembly reference error?
Hi, I am getting an error message while trying to create an array of Characters property. I am not clear how to resolve this issue.
using System.Web.Mvc;
using Treehouse.Models;
namespace Treehouse.Controllers
{
public class VideoGamesController : Controller
{
public ActionResult Detail()
{
var videoGame = new VideoGame()
{
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new Characters[]
{
new Characters(){"Mario"},
new Characters(){"Princess Peach"},
new Characters(){"Bowser"},
new Characters(){"Toad"},
new Characters(){"Yoshi"}
}
};
return View(videoGame);
}
}
}
@model Treehouse.Models.VideoGame
@{
ViewBag.PageTitle = "Video Game Detail";
}
<h1>@Model.Title</h1>
<h5>Description:</h5>
<div>@Model.Description</div>
<h5>Characters:</h5>
<div>
<ul>
@foreach (var character in Model.Characters)
{
<li>@character</li>
}
</ul>
</div>
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
1 Answer
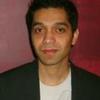
Mohammed Hossen
5,591 PointsNevermind, I figured out the solution.
var videoGame = new VideoGame()
{
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[5]
{
"Mario",
"Princess Peach" ,
"Bowser" ,
"Toad" ,
"Yoshi"
}
};