Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial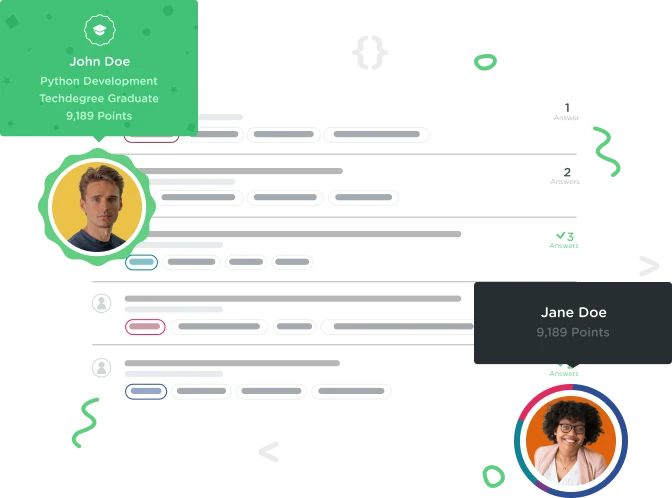
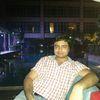
KnowledgeWoods Consulting
5,607 PointsHow to Restrict Duplicate Entries in Database?
I have built a form which accepts name,phone no. and message. It stores all the information in a database. I want that if a user enters same name and phone no. multiple times it echo "You are already registered".
7 Answers

Tobias Schulz
1,997 PointsSo change the SELECT Query like: "SELECT COUNT(*) FROM tablename WHERE username = ....."
With the COUNT(*) you will get the number of affected rows.
See this: http://stackoverflow.com/questions/2700621/php-pdo-num-rows

Tobias Schulz
1,997 PointsYou can do a SELECT on your Table with the entered username and phone no. If you getting a row back, the user is already registered.
Something like that:
SELECT * FROM tablename WHERE username = "mike" AND phone = "123456";
With mysql_num_rows() you can get how many rows you got with your query. mysql_num_rows()
Or you can set the username field as "UNIQUE" and you will get an error on INSERT.
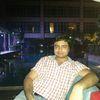
KnowledgeWoods Consulting
5,607 PointsBelow is my code. Please tell me how to proceed. I am using PDO connection.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; $email_body = ""; $email_body = $email_body . "Name: " . $name . "\n"; $email_body = $email_body . "Email: " . $email . "\n"; $email_body = $email_body . "Message: " . $message;
$db = new PDO("mysql:host=localhost;dbname=connection","root","");
$query = "INSERT INTO connect(name,email,message)VALUES('$name','$email','$message')"; $result = $db->query($query);
if($result) { echo "Successfully updated database"; }
}
?>
<h1>Contact</h1>
<p>I’d love to hear from you! Complete the form to send me an email.</p>
<form method="post" action="contact.php">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input type="text" name="name" id="name">
</td>
</tr>
<tr>
<th>
<label for="email">Email</label>
</th>
<td>
<input type="text" name="email" id="email">
</td>
</tr>
<tr>
<th>
<label for="message">Message</label>
</th>
<td>
<textarea name="message" id="message"></textarea>
</td>
</tr>
</table>
<input type="submit" value="Send">
</form>

Tobias Schulz
1,997 Points$selectFirstQuery = "SELET * FROM tablename WHERE username = $post_username AND phone = $post_phone;";
$queryResult = $db->query($selectFirstQuery);
$foundRows = $queryResult->num_rows;
if($foundRows >= 1) {
echo "You are already registered";
} else {
//Your Insert Code Here
}
This code is NOT tested. But it should help you to figure it out. You should looking for "mysql_real_escape_string" if your code is working. This will help you for sql injection.
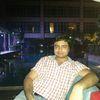
KnowledgeWoods Consulting
5,607 PointsNotice: Trying to get property of non-object
I read that num_rows is not a property of PDO class. Please help me...!!!
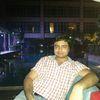
KnowledgeWoods Consulting
5,607 PointsI read that already. But still no help...:(
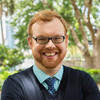
Andrew Chalkley
Treehouse Guest TeacherHave you tried using the UNIQUE
keyword as Tobias Schulz suggested?
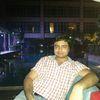
KnowledgeWoods Consulting
5,607 PointsAs far as i know, i can only make one column in database as unique. But i need two things to be unique. I have built a form which accepts name,phone no. and message. It stores all the information in a database. I want that if a user enters same name and phone no. multiple times it echo "You are already registered".
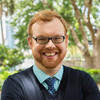
Andrew Chalkley
Treehouse Guest TeacherHere's an example of doing a unique on multiple columns:
ALTER TABLE table_name_here
ADD CONSTRAINT unique_constraint_name_phone UNIQUE (name,phone)
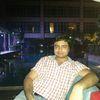
KnowledgeWoods Consulting
5,607 PointsThanks Andrew. That worked perfectly fine. But my need is a bit different. I want that when a person enters his information for example : he enter name=andrew and phone=12345 then second time name=andrew and phone=54321 then the entry should be saved in table. But when i make both the columns unique then this is not possible. I want combination of two things to be unique. Hope you understand.
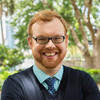
Andrew Chalkley
Treehouse Guest TeacherI don't know how you'd do it at the database level. You probably could do it at the application level depending on the error message you get back.
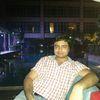
KnowledgeWoods Consulting
5,607 PointsMy need is a bit different. I want that when a person enters his information for example : he enter name=andrew and phone=12345 then second time name=andrew and phone=54321 then the entry should be saved in table. But when i make both the columns unique then this is not possible. I want combination of two things to be unique. Hope you understand.

Tobias Schulz
1,997 PointsTry to do a SELECT Query with the name and phone. If you getting a result, this pair of data already exists. If not you can do your insert.
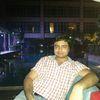
KnowledgeWoods Consulting
5,607 PointsFollowing is my code. please tell me what i am doing wrong. It is always showing "you are already registered". I think we had to check the no. of rows returned. Please Help.
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; $email_body = ""; $email_body = $email_body . "Name: " . $name . "\n"; $email_body = $email_body . "Email: " . $email . "\n"; $email_body = $email_body . "Message: " . $message;
$db = new PDO("mysql:host=localhost;dbname=connection","root","");
$query1="SELECT * FROM connect WHERE 'name'='$name' AND 'email'='$email'";
$res = $db->query($query1);
if ($res)
{
echo "you are already registered";
}
else {
$query = "INSERT INTO connect(name,email,message)VALUES('$name','$email','$message')";
$result = $db->query($query);
if($result) { echo "Successfully updated database"; } else {
echo"Not Updated";
}
}
} ?>
<h1>Contact</h1>
<p>I’d love to hear from you! Complete the form to send me an email.</p>
<form method="post" action="contact.php">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input type="text" name="name" id="name">
</td>
</tr>
<tr>
<th>
<label for="email">Email</label>
</th>
<td>
<input type="text" name="email" id="email">
</td>
</tr>
<tr>
<th>
<label for="message">Message</label>
</th>
<td>
<textarea name="message" id="message"></textarea>
</td>
</tr>
</table>
<input type="submit" value="Send">
</form>

Tobias Schulz
1,997 PointsTry a different SELECT QUERY:
SELECT COUNT(id) FROM mytable WHERE name='name' AND phone='phone'.
In your SQL answer you will find a field with the name COUNT. Check that number.
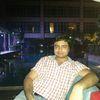
KnowledgeWoods Consulting
5,607 PointsIn your SQL answer you will find a field with the name COUNT. Check that number ?????
I don't get that. Can you please provide me with an example.

Tobias Schulz
1,997 PointsTry this with your select query and "count(*)"
$nRows = $pdo->query('select count(*) from blah')->fetchColumn(); echo $nRows;
tell us what you get returned by "echo $nRows;"
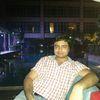
KnowledgeWoods Consulting
5,607 PointsI get my no. of rows i.e 2.

Tobias Schulz
1,997 PointsNice.
<?php
if($nRows >= 1) { echo "Already Registered"; } else { //insert here }
?>
And you´re done
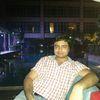
KnowledgeWoods Consulting
5,607 Points$nRows = $db->query("select count(*) from connect where name=$name AND email=$email")->fetchColumn();
Is this correct? It is returning 0 rows always

Tobias Schulz
1,997 PointsThis should be correct with PDO.
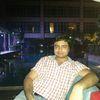
KnowledgeWoods Consulting
5,607 PointsThanks a lot Tobias Schulz for your help. It is finally working as i wanted. Thanks a ton.
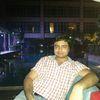
KnowledgeWoods Consulting
5,607 PointsThanks for your help....:)