Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial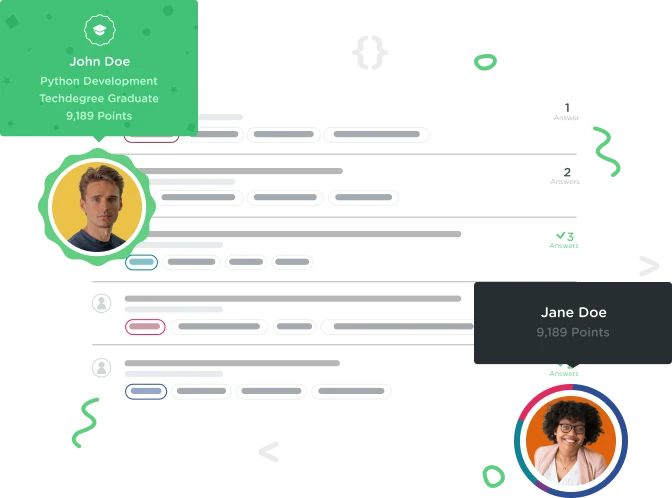
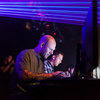
Adam Duffield
30,494 PointsHow to retrieve highest 4 values from an associative array in PHP?
Hi,
See my code below, I have many different car's with different views, I want to be able to retrieve the highest 4 views out of the array and display those cars to homepage to show off the most popular cars. Anybody able to assist me?
$cars[] = array( "ID" => "8", "Model" => "Fiesta", "Make" => "Ford", "Image" => "img/FordFiesta.jpeg", "MPG" => "60mpg", "Price" => "£2000", "Year" => "2007", "Mileage" => "55,829", "Views" => "295" );
function get_most_views(){ $cars = $GLOBALS["cars"]; $viewcount = count($cars["Views"]); return $viewcount; }
Thanks.
Adam
6 Answers
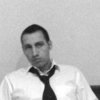
Alex Heil
53,547 Pointshey adam, there was a code chellenge with a function that returned the results with the most likes. I guess that will work absolutely fine for you as well when you change the "likes" into "views".
here's the code from the challenge - hope that helps ;)
function get_all_flavors() {
$flavors = array(
array("name" => "Vanilla", "likes" => 312),
array("name" => "Cookie Dough", "likes" => 976),
array("name" => "Peppermint", "likes" => 12),
array("name" => "Cake Batter", "likes" => 598),
array("name" => "Avocado Chocolate", "likes" => 6),
array("name" => "Jalapeno So Spicy", "likes" => 3),
);
return $flavors;
}
function get_flavors_by_likes($number) {
$all = get_all_flavors();
$total_flavors = count($all);
$position = 0;
$popular = $all;
usort($popular, function($a, $b) {
return $b['likes'] - $a['likes'];
});
return array_slice($popular, 0, $number);
}

Charles Gray
19,470 Pointshave you tried sorting the array?

Charles Gray
19,470 Pointsoh never mind that response
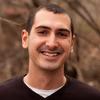
tihomirvelev
14,109 PointsMaybe it's not the best way but it will work.
<?php
$cars = array(
1 => array(
"Model" => "Nissan RX",
"Views" => 200),
2 => array(
"Model" => "Mazda Z",
"Views" => 300),
3 => array(
"Model" => "Mercedes G",
"Views" => 400),
4 => array(
"Model" => "Subaru",
"Views" => 100),
5 => array(
"Model" => "Cadillac",
"Views" => 500),
6 => array(
"Model" => "Renault",
"Views" => 50),
7 => array(
"Model" => "Renault",
"Views" => 550)
);
function highestViews() {
$cars = $GLOBALS["cars"];
$return_highest = array();
$views = array();
/*Pushing into the $views array only
*the views numbers
*/
foreach($cars as $car) {
array_push($views, $car['Views']);
}
$total = count($views);
$counter = 1;
$for_show = 4;
/* Setting up a while loop.
* $total - $for_show gives us
* the number of cars to be removed.
* That way in the array are staying
* only the wanted cars.
*/
while ($counter <= $total - $for_show) {
$counter++;
/*search for the min value of views
and removing it.*/
$key = array_search(min($views), $views);
unset($views[$key]);
}
/*Comparing each value in the $views array
* with each "Views" in the $cars array.
* If equal, pushes that car into a new array
*/
foreach ($cars as $car) {
foreach($views as $key => $value) {
if ($car["Views"] == $value) {
array_push($return_highest, $car);
}
}
}
return $return_highest;
}
/* you can check if it works with:
* echo "<pre>";
* var_dump(highestViews());
*/
?>
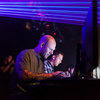
Adam Duffield
30,494 PointsHey tihomervelev,
Output is this...
array(4) { [0]=> array(9) { ["ID"]=> string(1) "3" ["Model"]=> string(2) "A1" ["Make"]=> string(4) "Audi" ["Image"]=> string(14) "img/AudiA1.jpg" ["MPG"]=> string(5) "60mpg" ["Price"]=> string(6) "£2000" ["Year"]=> string(4) "2007" ["Mileage"]=> string(6) "55,829" ["Views"]=> int(2384) } [1]=> array(9) { ["ID"]=> string(1) "4" ["Model"]=> string(3) "107" ["Make"]=> string(6) "Peugot" ["Image"]=> string(17) "img/Peugot107.jpg" ["MPG"]=> string(5) "60mpg" ["Price"]=> string(6) "£2000" ["Year"]=> string(4) "2007" ["Mileage"]=> string(6) "55,829" ["Views"]=> int(298) } [2]=> array(9) { ["ID"]=> string(1) "5" ["Model"]=> string(5) "Civic" ["Make"]=> string(5) "Honda" ["Image"]=> string(18) "img/HondaCivic.jpg" ["MPG"]=> string(5) "60mpg" ["Price"]=> string(6) "£2000" ["Year"]=> string(4) "2007" ["Mileage"]=> string(6) "55,829" ["Views"]=> int(2293) } [3]=> array(9) { ["ID"]=> string(1) "7" ["Model"]=> string(3) "Rx8" ["Make"]=> string(5) "Mazda" ["Image"]=> string(16) "img/MazdaRx8.jpg" ["MPG"]=> string(5) "60mpg" ["Price"]=> string(6) "£2000" ["Year"]=> string(4) "2007" ["Mileage"]=> string(6) "55,829" ["Views"]=> int(300) } }
Can't quite understand why, Thanks for trying though!
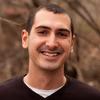
tihomirvelev
14,109 PointsYou are getting exactly what you have to - an associative array with four nested arrays, each one representing one of the four cars with highest views.
Now you have to loop through this array. You will call the needed elements through their keys and values and display the data where you want on your page.
It's actually a very long story to do it here and it cannot happen. I recommend you to take the Randy's course Enhancing a Simple PHP Application (if you have not done so far): http://teamtreehouse.com/library/enhancing-a-simple-php-application-2
Good luck!
Adam Duffield
30,494 PointsAdam Duffield
30,494 PointsHey,
I gave it a go and played with the code quite a bit but this isn't quite what I'm after, my function doesn't have any arguments, I just have about 10 cars with a different amount of views and I need to return the top 4 cars in terms of views. It's pretty much the same as this but I don't have anything I can use for the $number variable.