Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial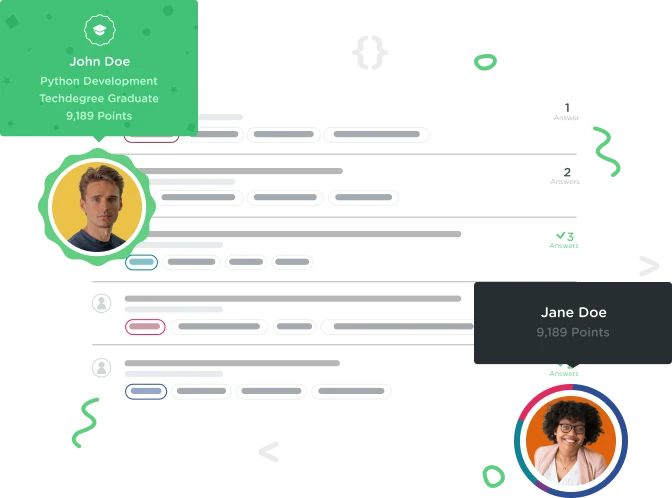
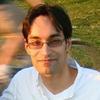
Rizwan Khan
24,089 PointsHow to return a new map in Go?
The HalfPriceSale function should receive a map with product name strings as its keys, and corresponding prices as its values, like this:
{"OCKOPROG": 89.99, "ALBOÖMME": 129.99, "TRAALLÅ", 49.99}
It should return a new map with all the same keys, but all the corresponding values divided in half, like this:
{"OCKOPROG": 44.995, "ALBOÖMME": 64.995, "TRAALLÅ", 24.995}
Here is my code:
package sales
func HalfPriceSale(prices map[string]float64) map[string]float64 {
for _, v := range prices {
return v/2
}
}
2 Answers
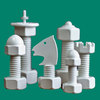
Steven Parker
231,268 PointsYou'll need to build a new map in your function, then you can return it. Also, you won't want to return inside the loop, you'll want to wait until the loop finishes going through the entire data.
There's an example of building up a map in the first half of the Maps video.

Tony Brackins
28,766 PointsI did the same but returned prices and didn't declare new var.
Rizwan Khan
24,089 PointsRizwan Khan
24,089 PointsTook me some time, but finally figured it out. Thanks for your help.