Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial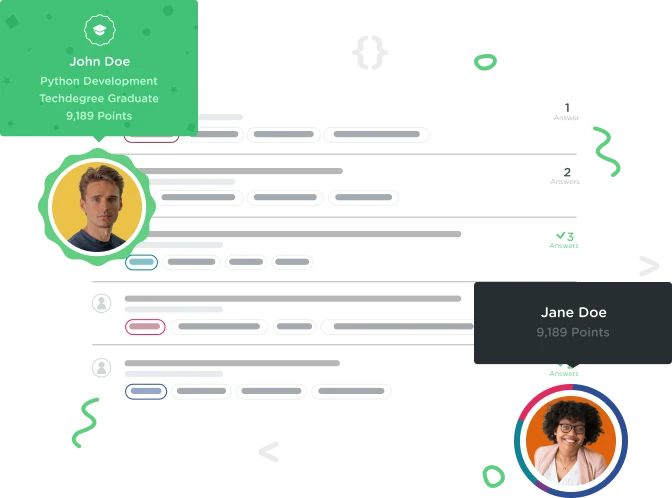

Jason Zhang
1,157 PointsHow to return a tuple
Hi all,
I am stuck by this question, the question asks to modify the function so that it returns tuple instead of string. But I don't get how I could do that? There's no type Tuple right? And am I suppose to return the name of the tuple of each individual elements of the tuple?
Thanks very much.
Kind Regards, Jason
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
4 Answers
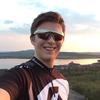
Erik Uusitalo
8,096 PointsThe "return type" for a tuple is (type, type). In this case both variables is of type String. So it should look like this...
func greeting(person: String) -> (greeting: String, language: String){
let language = "English"
let greeting = "Hello \(person)"
}
And when you have told the function what it is supposed to return. You just have to put the variables in a Tuple and then return it.
func greeting(person: String) -> (greeting: String, language: String){
let language = "English"
let greeting = "Hello \(person)"
//Saving tuple to variable and returning it
let t = (greeting , language)
return t
}
Or you could write it a bit shorter like this...
func greeting(person: String) -> (greeting: String, language: String){
let language = "English"
let greeting = "Hello \(person)"
//Returning the tuple directly
return (greeting , language)
}
Hope you find this helpful. Best Regards, Erik

Chris Bernardi
3,994 PointsErik clearly you type faster than me :)
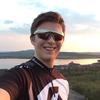
Erik Uusitalo
8,096 PointsThere can never be too many answers :).

Chris Bernardi
3,994 Points@Jason here's the answer:
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
The tuple part is what follows after the -> Here you are defining that this function returns two items both being strings (greeting & language). What this means is that later when using the function greeting(), you can access both the greeting string and language string idividually
var result = greeting("Tom") // (.0 "Hello Tom", .1 "English")
println(result.language) // "English"
Whenever I get stuck, I go back and watch the videos and redo the playgrounds. And then, I ask here :)

Jason Zhang
1,157 PointsThanks Chris, Erik! Do the names of the tuples matter? I've changed the names to tuple1 and tuple2 for the return type(see below) and I could see that it works too
func greeting(person: String) -> (tuple1: String, tuple2: String){
let language = "English"
let greeting = "Hello \(person)"
//Returning the tuple directly
return (greeting , language)
}
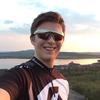
Erik Uusitalo
8,096 PointsNo it doesn't mather, you can name it whatever you want.
But the name is like a "variable" so it should have a "description" of whats inside the tuple at that position, because it is this name you will use when you refer to that position like this...
func greeting(person: String) -> (tuple1: String, tuple2: String){
let language = "English"
let greeting = "Hello \(person)"
//Returning the tuple directly
return (greeting , language)
}
//In you case it will look like this
result = greeting("Jason")
result.tuple1 //this refers to "greeting"
result.tuple2 //this refers to "language"
Instead of naming them tuple1 and tuple2 you should name them something like greeting and language like this...
func greeting(person: String) -> (greeting: String, language: String){
let language = "English"
let greeting = "Hello \(person)"
//Returning the tuple directly
return (greeting , language)
}
//Another way of doing it
result = greeting("Jason")
result.greeting //this refers to "greeting"
result.language //this refers to "language"
Like you see this makes more sense.

Jason Zhang
1,157 PointsGot it, thanks Erik!
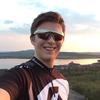
Erik Uusitalo
8,096 Pointsi added some stuff at the end. And no problem!
Jason Zhang
1,157 PointsJason Zhang
1,157 PointsThis is the original Treehouse code question:
//Currently our greeting function only returns a single value. Modify it to return both the greeting and the language as a tuple. Make sure to name each item in the tuple: greeting and language. We will print them out in the next task.