Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial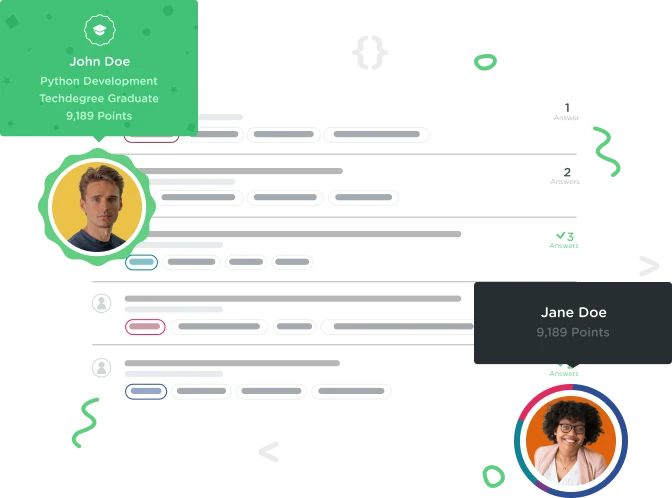

Matt Smith
878 PointsHow to return a tuple containing two values?
What do I need to change to fix the error? Can someone recap for me too what a tuple is and how it relates to Doubles.
// Enter your code below
func getTowerCoordinates(location: String) {
switch location {
case "Eiffel Tower": (48.8582, 2.2945)
case "Great Pyramid": (29.9792, 31.1344)
case "Sydney Opera House": (33.8587, 151.2140)
default: 0.0
}
return
}
getTowerCoordinates("Eiffel Tower")
4 Answers
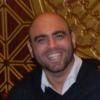
Mohamed Moukhtar
Courses Plus Student 6,151 PointsA tuple is a group of zero or more values represented as one value. For example a tuple of ("Matt", "Smith") holds your first and last name. You can access the inner values using the dot(.) notation followed by the index of the value For example: var person = ("Matt", "Smith") // a tuple var firstName = person.0 // Matt var lastName = person.1 // Smith
Tuples are value types and it can hold values of any type like Double, Int, or String
And here is a fix for your code
func getTowerCoordinates(location: String) -> (latitude: Double, longitude: Double) {
switch location {
case "Eiffel Tower": return (48.8582, 2.2945)
case "Great Pyramid": return (29.9792, 31.1344)
case "Sydney Opera House": return (33.8587, 151.2140)
default: return (0,0)
}
}
let cooridnate = getTowerCoordinates("Eiffel Tower") cooridnate.latitude cooridnate.longitude
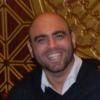
Mohamed Moukhtar
Courses Plus Student 6,151 PointsI am so sorry for the inconvenience, I didn't check the challenge before submitting my reply.
try to copy the following code and it will work:
func getTowerCoordinates(location: String) -> (Double, Double) { switch location { case "Eiffel Tower": return (48.8582, 2.2945) case "Great Pyramid": return (29.9792, 31.1344) case "Sydney Opera House": return (33.8587, 151.2140) default: return (0,0) } }
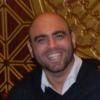
Mohamed Moukhtar
Courses Plus Student 6,151 PointsThe difference between the two codes are: 1- In the second code I didn't use a named tuple (to comply with the challenge requirement) 2-In the second code I removed the coordinate constant
but both codes will work with you on Xcode Playground.
I hope that I helped you.

Matt Smith
878 PointsThank you, trying to pass the challenge in the mean time certainly got me familiar with tuples.

Tyron Spencer
1,489 PointsHi, Mohamed Moukhtar .. Just wanted to thank you, this helped me also

Antonio Moreda
2,304 PointsThis worked for me on the XCode playground giving the correct result but didn't work on the Challenge:
func getTowerCoordinates(location: String) -> (lat: Double, lon: Double) {
let lat = Double()
let lon = Double()
switch location {
case "Eiffel Tower": return(48.8582, 2.2945)
case "Great Pyramid": return(29.9792, 31.1344)
case "Sydney Opera House": return(33.8587, 151.2140)
default: return(0,0)
}
}
let result = getTowerCoordinates("Eiffel Tower")
result.lat
result.lon

Antonio Moreda
2,304 PointsWell this Challenge took forever but i finally got it to work. All I needed to do was eliminate the names for the return values.
func getTowerCoordinates(location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": return(48.8582, 2.2945)
case "Great Pyramid": return(29.9792, 31.1344)
case "Sydney Opera House": return(33.8587, 151.2140)
default: return(0,0)
}
}
Matt Smith
878 PointsMatt Smith
878 PointsThanks for the explanation of Tuples. But, I've tried copying your exact reply into the editor and it isn't letting me progress.