Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial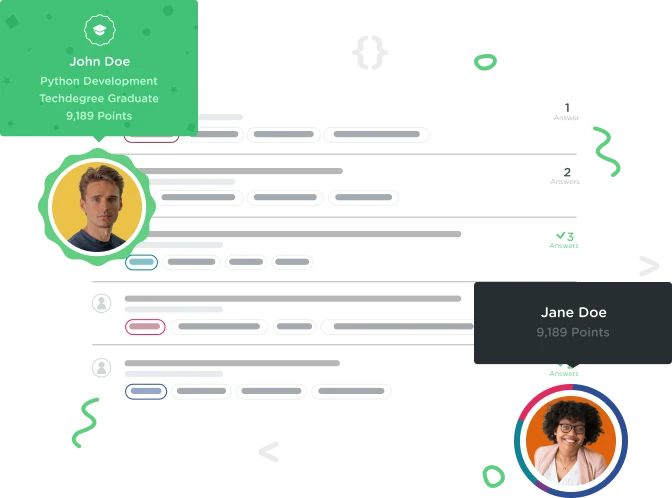

Viet Nguyen
564 PointsHow to return the function ?
How do I return the function from the output?
import math
def square(number):
print(number)
print("A **square* is mulitiplied by itself?")
return number * 3
square(num_square / function_total)
num_square = int(input("What is the value square?"))
function_total = float(input("What is the total?"))
the_sum = square(num_square, funtion_total)
3 Answers
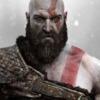
boi
14,242 PointsI don't understand what are you trying to get out of this code, it seems you are trying yo divide the input values and then multiply them with 3 and then return the value?.... anyways there are a few syntax
problems with your code
1) Let's analyze the error messages you are getting, the first error message is in the fifth line of code.
import math
def square(number):
print(number)
print("A **square* is mulitiplied by itself?")
return number * 3 # HERE is the first error you are getting
in the REPL you get a message "SyntaxError: 'return' outside function" which makes sense since your return
is indented outside the function, solve this by indenting the return
into the function.
2) You have a spelling error on line number 9 (or the last line) you spelled "funtion" instead of "function". ALSO why does this line of code even exist? delete the last line of code or line number 9
3) Your function call is at the wrong line, it should be at the end of your code, try replacing line number 9 with line number 6 like this;
import math
def square(number):
print(number)
print("A **square* is mulitiplied by itself?")
return number * 3
square(num_square / function_total) # wrong line for this line of code, it should be at last of your code
num_square = int(input("What is the value square?"))
function_total = float(input("What is the total?"))
the_sum = square(num_square, funtion_total) # delete this line of code and replace it with line number 6
#fixed code here below
import math
def square(number):
print(number)
print("A **square* is mulitiplied by itself?")
return number * 3
num_square = int(input("What is the value square?"))
function_total = float(input("What is the total?"))
square(num_square / function_total)
And finally, your main question of how to return the value, just use the print
function on your function call like this;
print(square(num_square / function_total))

Viet Nguyen
564 Pointsok thanks a lot that was helpful.
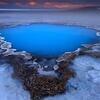
Brock Goodrich
991 PointsI found on the Python website myriads of math functions and other python codes that could be used as a reference chart. The previous video did not go in depth. The answer to returning the squared function could also be
<def square(number):
solution = pow(number, 2)
return solution>
Exclude the <> in the beginning and end because I do not fully understand how to fix that.
Justin Cox
12,123 PointsJustin Cox
12,123 PointsAre you just trying to make a function the squares a number passed into it? If so, you could just use something like this:
or as a full example:
You won't need to import math unless you use one of its functions, so I removed that. I also wasn't really sure what
function_total
is, or the division you have on line 6, so I didn't include any of that, but this code should hopefully get you closer to what you're trying to achieve. Good luck!