Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial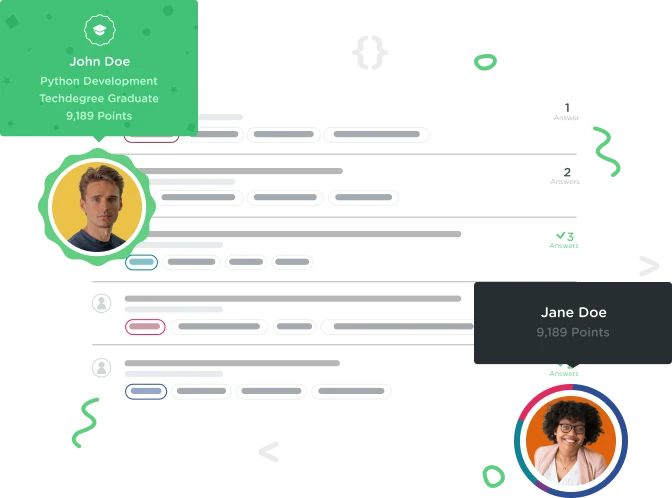
Kai Aldag
Courses Plus Student 13,560 Pointshow to run a ruby program
hey guys,
so for the extra credit in the math badge it says make a program that asks the user for input 2 numbers then add them together. anyways i build i version of it and i can't get it to run, it says that undefined method 'math' in calculator (noMethodError). anyways here's my code.
class Calculator
def math
print "please enter the first number"
number1 = gets
print "please enter the secound number"
number2 = gets
answer = #{number1} + #{number2}
puts = "#{answer}"
end
end
Calculator.math
please help, thanks, Kai.
1 Answer
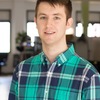
Ethan Lowry
Courses Plus Student 7,323 PointsTry:
calc = Calculator.new
calc.math
Unless you specifically define a method as a class method, that method should be called on an individual instance of the class, not the class itself.
Kai Aldag
Courses Plus Student 13,560 Pointsok thank you, and second i now can't get it to run, i can input my 2 number but then the program ends i modified my code so here it is
class Calculator
def math
print "please enter the first number"
number1 = gets
print "#{number1}"
print "please enter the secound number"
number2 = gets
print "#{number2}"
answer = #{number1} + #{number2}
print = "#{answer}"
end
end
calc = Calculator.new
calc.math
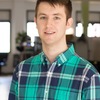
Ethan Lowry
Courses Plus Student 7,323 PointsThe line where you are trying to assign your answer variable is wrong - you only use the hash symbols like that when using string interpolation a.k.a. inserting variable values within strings.
What you want to do there is simply:
answer = number1 + number2
puts answer
Note that you may also have to convert your numbers to integers (whole numbers) or floats (decimal numbers) using to_i or to_f respectively, since you can't perform math operations on strings.
As a final note, similar to what I said above, the use of string interpolation is unnecessary on all of the lines where you are simply printing the value of a variable without any other string content. You should simply put this instead:
puts number1
Hope that all helps.
Kai Aldag
Courses Plus Student 13,560 Pointsok thanks a lot but now i have one more issue, when i run the program it outputs the first number then the second, they're not not being added together. once again here's my code.
class Calculator
def math
print "please enter the first number"
number1 = gets
puts number1
print "please enter the secound number"
number2 = gets
puts number2
answer = number1 + number2
puts answer
end
end
calc = Calculator.new
calc.math
thanks, once again, for the help Ethan Lowry
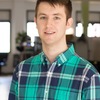
Ethan Lowry
Courses Plus Student 7,323 PointsYou might need to convert your variables from strings to numbers as I mentioned before. What happens if you instead do the following:
answer = number1.to_i + number2.to_i
Where "to_i" converts a string to an integer. If you're using numbers with decimal spaces then use to_f for converting to float instead.
Kai Aldag
Courses Plus Student 13,560 Pointswow thanks so much it works like a charm.
thanks for the help, Kai.
Ethan Lowry
Courses Plus Student 7,323 PointsEthan Lowry
Courses Plus Student 7,323 PointsYou might need to convert your variables from strings to numbers as I mentioned before. What happens if you instead do the following:
answer = number1.to_i + number2.to_i
Where "to_i" converts a string to an integer. If you're using numbers with decimal spaces then use to_f for converting to float instead.