Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial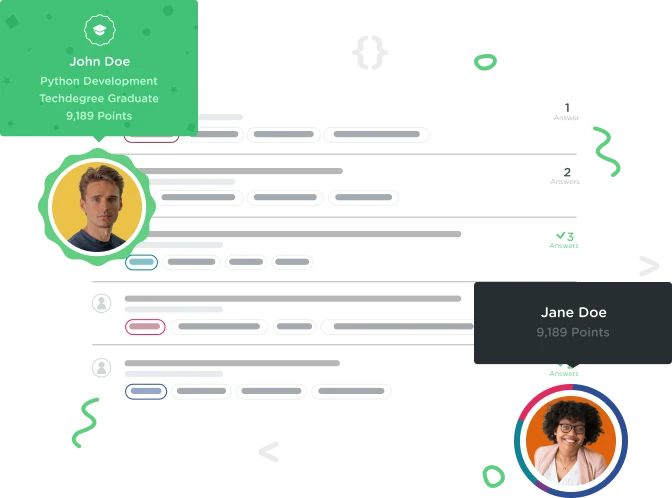

Robert Baucus
3,400 PointsHow to run morse.py (to test it like the challenge does)
I was able to pass the morse.py challenge (by the skin of my teeth) but I am not really confident with Classes and was experimenting with them to solidify my knowledge. Messing around in the shell, I realized that I don't even know how to run the program so that morse turns the pattern, "." "." "." into "dot" "dot" "dot".
Here is the code that I wrote:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
pattern = []
for i in pattern:
if i == ".":
pattern.append("dot")
elif i == "_":
pattern.append("dash")
return "-".join(pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
It seems to work ok in the workspace on treehouse, but I can't figure how to run this on my own computer. Here is a printout of what I have tried (Win10):
>>> from morse import Letter,S
>>> s = S()
>>> print(s)
>>> s = S(Letter)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: __init__() takes 1 positional argument but 2 were given
>>> s = Letter()
>>> s
<morse.Letter object at 0x000001028BC8C780>
>>> s.pattern
>>> print(s.pattern)
None
>>> print(s.__str__)
<bound method Letter.__str__ of <morse.Letter object at 0x000001028BC8C780>>
>>>
1 Answer
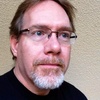
Chris Freeman
Treehouse Moderator 68,423 PointsThe Letter.__str__
method is using the local variable pattern
in the for
loop iterable instead of the attribute self.pattern
. By using the local variable pattern
there is nothing to loop over since it was just initialized to the empty list.