Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial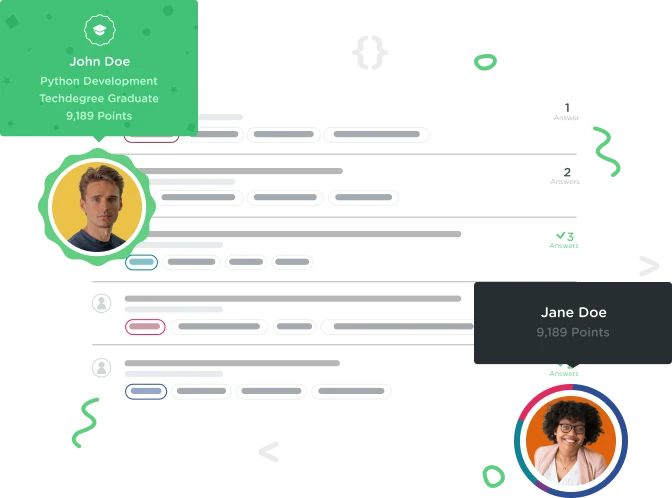

Nick Burton
7,387 PointsHow to run setInterval() with dynamic delay value
I understand how I would generate a random number and pass it into the setInterval function to consistently "tick" with the generated delay in between each tick. But, what if I wanted to have a dynamic delay for each tick? Meaning the time gap between each tick would be different every time. I tried to pass a function to generate the delay but it seems to only be executed once. Here's my code that doesn't work:
const randNumGenerator = () => (Math.floor(Math.random() * 5) + 1) * 1000;
setInterval(() => {
console.log('tick');
}, randNumGenerator());
Can someone explain to me how to go about this or point me in the right direction? Thanks!
2 Answers
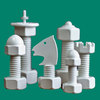
Steven Parker
229,744 PointsThe random generator is only called once, and then the "setInterval" function just repeats that setting over and over. But you can set fresh conditions for each tick by using a wrapper function to set a single timeout and then have it call itself when the tick occurs to set up the next one:
function randomTick() {
setTimeout(() => {
console.log("tick");
randomTick(); // set up the next tick
}, randNumGenerator());
}
randomTick(); // start the first tick
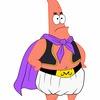
<noob />
17,062 PointsSteven Parker i would like to know if there is a way to, the closet thing i got is to set it evreytime the page load to have a diff delay
function tickClock() {
clockSection.textContent = getTime();
}
tickClock();
setInterval(tickClock,Math.random() * 4000);
Nick Burton
7,387 PointsNick Burton
7,387 PointsWoah, that's clever! Thank you for taking the time to respond with that example.