Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial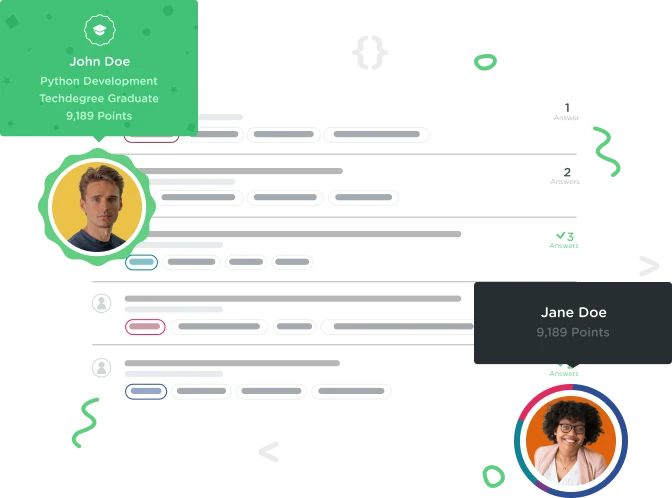
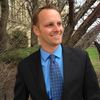
robert cioffi
3,466 PointsHow to save addButton additions to Master View in Master-Detail Template after quitting app
I have a master-detail program that I add items through a UIAlert to the master list, but when the app quits, the items I added are gone, how can I save the values I added? How would I change to the code below to do this? I've heard you can save to the plist, but I don't know how to use that functionality. Thanks!
If you are curious how I change the NSDate to a pop up AlertView to add any value you want look here:
-
(void)viewDidLoad { [super viewDidLoad];
self.navigationItem.leftBarButtonItem = self.editButtonItem;
UIBarButtonItem *addButton = [[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemAdd target:self action:@selector(insertNewObject:)]; self.navigationItem.rightBarButtonItem = addButton; }
(void)insertNewObject:(id)sender //modified to pop up alertView see: http://stackoverflow.com/questions/11163341/how-do-i-replace-the-date-with-a-writable-title { UIAlertView *getTitle = [[UIAlertView alloc] initWithTitle:@"Add Search Keyword" message:nil delegate:self cancelButtonTitle:@"Add" otherButtonTitles:nil]; getTitle.alertViewStyle = UIAlertViewStylePlainTextInput; [getTitle show]; }
(void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex { if (!_objects) { _objects = [[NSMutableArray alloc] init]; } NSString * userEnterThisString = [[alertView textFieldAtIndex:0] text]; [_objects insertObject:userEnterThisString atIndex:0]; NSIndexPath *indexPath = [NSIndexPath indexPathForRow:0 inSection:0]; [self.tableView insertRowsAtIndexPaths:[NSArray arrayWithObject:indexPath] withRowAnimation:UITableViewRowAnimationAutomatic]; self.keepValue = userEnterThisString; }
1 Answer
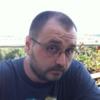
Robert Bojor
Courses Plus Student 29,439 PointsHi Robert,
Here's a bit of code to help you out with the problem you are facing...
// To save the data to a file you can do this...
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *addedItems = [[paths objectAtIndex:0] stringByAppendingPathComponent:@"addedItems.dta"];
// The file name and extension can be changed at will
[_objects writeToFile:addedItems atomically:YES];
// To read it back you can do this
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
if ([paths count] > 0) {
NSString *addedItems = [[paths objectAtIndex:0] stringByAppendingPathComponent:@"addedItems.dta"];
BOOL fileExists = [[NSFileManager defaultManager] fileExistsAtPath:addedItems];
if (fileExists) {
_objects = [NSArray arrayWithContentsOfFile:addedItems];
} else {
// Notify the user the file doesn't exist or try to load it from a cached resource.
}
}
If you want to cache a file I believe you can use SAMCache, a nice CocoaPod written by one of the teachers here at Treehouse, Sam Soffes.
robert cioffi
3,466 Pointsrobert cioffi
3,466 Pointsexcellent response Robert, that works like a charm! For anyone else curious I added the save data to file portion of the code to the end of the AlertView method, and I added the read it back in the viewDidLoad (both in the Master View)
Here is final code if you are interested: