Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial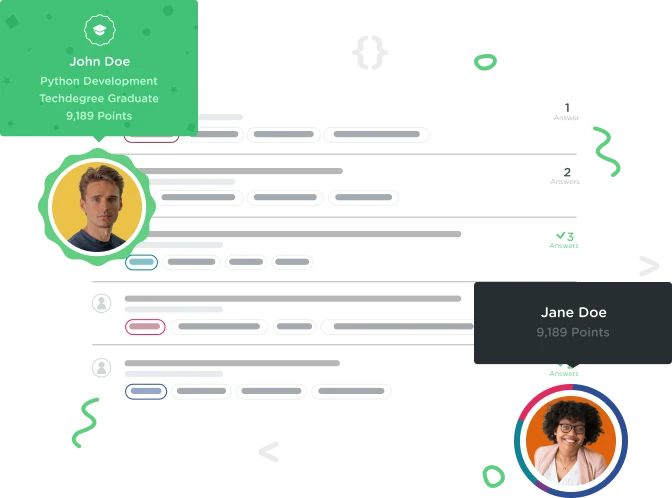
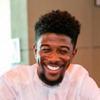
James Carter
14,580 PointsHow to search for the first instance of a string in an array?
I am currently trying to build a basic seat reservation system using javascript. How would i go about finding the first instance of the string "red" in my seats array? Also, once found, i wish to have that value displayed in my html inbox Here is my current failed attempt. The input box only seems to show seat #9, even after change the color from red to grey with the mouse.
<html>
<head>
<title>Seat Reservations</title>
<script type="text/javascript">
seats = ["red", "red", "red", "red", "red", "red", "red", "red", "red", "red"];
function changeColor(n) {
if (seats[n] == "red") {
document.getElementById("seat" + n).src = "./grey.png";
seats[n] = "grey";
}else {
document.getElementById("seat" + n).src = "./red.png";
seats[n] = "red";
}
}
function findSeat(n) {
console.log ("Hello")
for (i=0;i<seats.length;i++){
if (seats[i] == "red")
{document.getElementById("text").value = "the first available seat is" + i}
else
{document.getElementById("text").value = "no seats available"}
}
}
</script>
</head>
<center> <h1> Seat Reservation System </h1> </center>
<hr>
<body>
<img id="seat1" src="./red.png" alt="" onClick="changeColor(1);">
<img id="seat2" src="./red.png" alt="" onClick="changeColor(2);">
<img id="seat3" src="./red.png" alt="" onClick="changeColor(3);">
<img id="seat4" src="./red.png" alt="" onClick="changeColor(4);">
<img id="seat5" src="./red.png" alt="" onClick="changeColor(5);">
<img id="seat6" src="./red.png" alt="" onClick="changeColor(6);">
<img id="seat7" src="./red.png" alt="" onClick="changeColor(7);">
<img id="seat8" src="./red.png" alt="" onClick="changeColor(8);">
<img id="seat9" src="./red.png" alt="" onClick="changeColor(9);">
<br></br>
<input type="button" id="button" value="Find Seats" onClick="findSeat();">
<input type="text" id="text" size= "50" value="">
</body>
</html>

james white
78,399 PointsHi James, I'm not an expert in javascript (still just learning) but if you are looking to debug this this I suggest using System.out.println to trace out the value of seat[i] after the line: for (i=0;i<seats.length;i++)
In case you don't know how to do this I saw some other "Loop airplane seating reservations" code online that show how to do this: http://www.coderanch.com/t/642227/java/java/Loop-airplane-seating-reservations-seats
Good Luck!
2 Answers
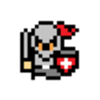
Michael Jurgensen
8,341 PointsHi James,
This will get the first instance of the string "red" :
var seats = ["blue", "red", "red"];
function isRed()
{
for(index in seats)
{
if(seats[index] === "red")
return index;
}
return -1;
}
alert(isRed());
I hope this helps.
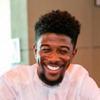
James Carter
14,580 PointsThanks for all the help guys! Here's something I came up with somewhat based off of Michael's suggested code. It seems to be working pretty well so far. However, now I'm having trouble getting the the current first available seat to display as green. Here's the updated code :
<html>
<head>
<title>Intro to Javascript</title>
<center> <h1> Reserving Seats </h1> </center>
<hr>
<script type="text/javascript">
seats=["red", "red", "red", "red", "red", "red", "red", "red", "red"];
function changeColor(n){
if (seats[n]=="grey"){
document.getElementById("seat"+n).src="./red.png";
seats[n]="red";
}
else{
document.getElementById("seat"+n).src="./grey.png";
seats[n]="grey";
}
}
function findFirstRed() {
for (var i = 0; i < seats.length; i++) {
var current_seat = document.getElementById("seat" + i);
if (current_seat.src.indexOf("red.png") >0) {
document.getElementById("textbox").value = "Seat " + i + " is free!";
current_seat.src = "./green.png";
var answer = prompt("Will you take this seat? (Y/N)");
if (answer === "y" || answer === "Y") {
current_seat.src = "./grey.png";
document.getElementById("textbox").value = "Seat " + i + " is taken.";
return;
} if (answer === "n" || answer === "N") {
current_seat.src = "./grey.png";
return;
}else {
current_seat.src = "./red.png";
}
}
}
document.getElementById("textbox").value = "No other seats free";
}
</script>
</head>
<body>
<img id="seat0" src="./red.png" alt="" onClick="changeColor(0);">
<img id="seat1" src="./red.png" alt="" onClick="changeColor(1);">
<img id="seat2" src="./red.png" alt="" onClick="changeColor(2);">
<img id="seat3" src="./red.png" alt="" onClick="changeColor(3);">
<img id="seat4" src="./red.png" alt="" onClick="changeColor(4);">
<img id="seat5" src="./red.png" alt="" onClick="changeColor(5);">
<img id="seat6" src="./red.png" alt="" onClick="changeColor(6);">
<img id="seat7" src="./red.png" alt="" onClick="changeColor(7);">
<img id="seat8" src="./red.png" alt="" onClick="changeColor(8);">
<br>
<input type="button" id="totalbutton" onClick="findFirstRed()" value="Find a seat!">
<br>
<input type="text" id="textbox" value="" size=30>
</body>
</html>
Any clue on where I went wrong?
Thanks,
James
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherJames;
Would love to see your solution to this once you get it working.
Ken