Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial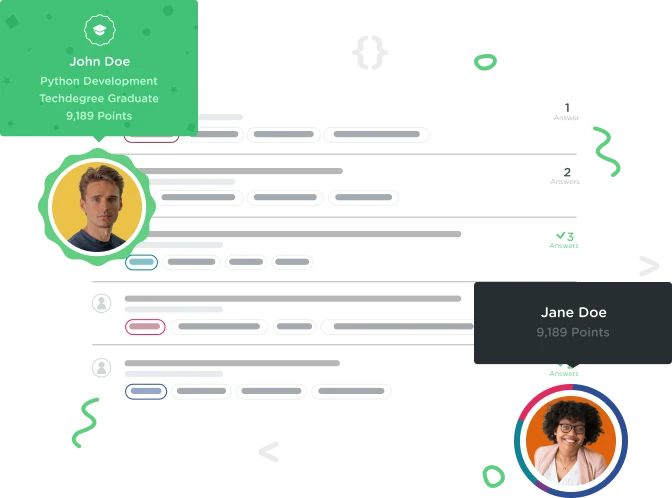
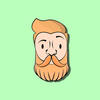
Jacob Kortbeek
10,929 Pointshow to Search with REST API, Ajax
I am trying to search my own mongodb for the occupation of signed up users and the return the name, occupation and email of the users.
Express (app.js)
const User = require(../models/user.js);
app.get('/search', (req, res, next) => {
User.find({occupation: req.body.search}, {_id: 0, name: 1, email: 1, occupation: 1, logInTime: 1}, {sort: {logInTime: -1}}, function (err, User) {
if(err) {
return next(err)
} else {
res.json(User);
};
});
});
Ajax
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
var users = JSON.parse(xhr.responseText);
var htmlStatus = '';
for(let i = 0; i < users.length; i ++){
htmlStatus += '<div class="recentOnline"><table><tbody><tr><td><div class="displayPic"><img src="/static/css/assets/wallpaper.png"></div></td><td>';
htmlStatus += '<p>';
htmlStatus += users[i].name;
htmlStatus += '</p>';
htmlStatus += '<p>';
htmlStatus += users[i].occupation;
htmlStatus += '</p>';
htmlStatus += '<p>';
htmlStatus += users[i].email;
htmlStatus += '</p>';
htmlStatus += '</td></tr></tbody></table></div>';
}
document.getElementById("searchResult").innerHTML = htmlStatus;
}
};
xhr.open('GET', '/search');
xhr.send();
Pug
extends index.pug
block content
.container-colums
.colum
h2 Profile
.profile
.displayProfileImg
img(src="/static/css/assets/wallpaper.png")
p #{name}
p #{occupation}
p #{email}
.colum
h2 Last Online
#recent
.colum
.searchBar
form(method="GET" action="search")
input(name="occupation" type="text" placeholder="Search")
button(type='submit' id='searchSubmit') Search
#searchResult
I have linked all the files necessary and can get the json response if i change the get to post, but it redirects me. it works if i directly put the occupation in the code but now when i try to get it form the search field.
if you need me to post the entire document i can.
any help please been trying to do this for weeks
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherGET will put the form contents into a query string. To access the query string use req.query.NAME_OF_PROPERTY. You can see the reference to the documentation on req.query here.