Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial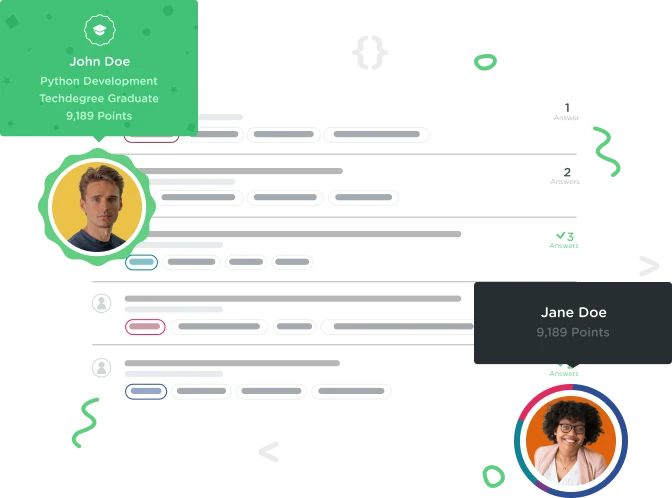
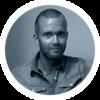
Daniel Fitzhugh
9,715 PointsHow to "select all <li> elements with getElementsByTagName and use length of the collection to access the last element"?
Around 1:50 in the "Removing Nodes" video the teacher says:
"Now for the list item element we'll need to select the last element in the list. And we can do this a number of different ways. We could select all the <li> elements with getElementsByTagName for example then find the length of the collection and use that to access the last element. That would be a perfectly valid approach but I'm gonna show you another way using query selector and the CSS pseudo class the last child...."
I wanted to see if I could work out how to do the first method he mentions above, but my knowledge of selectors syntax isn't good enough.
Given the following code, does anyone know how to accomplish what the teacher proposes above ?
removeItemButton.addEventListener( "click", () => {
let parentList = document.querySelector("ul#fullList");
let removedListItem = document.querySelector("ul#fullList li:last-child");
parentList.removeChild(removedListItem);
});
2 Answers
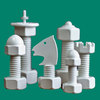
Steven Parker
232,176 PointsHere's a version doing what was described. As you can see, it's not as elegant and requires an extra step:
removeItemButton.addEventListener( "click", () => {
let parentList = document.querySelector("#fullList");
let allItems = parentList.getElementsByTagName("li"); // select all the "li" elements
let removedListItem = allItems[allItems.length - 1]; // use the length to access the last one
parentList.removeChild(removedListItem);
});
Also, just FYI, you don't need to select using a tag name and an id value, since id's must always be unique. So just "#fullList" is as good as "ul#fullList" for identifying the element. The longer selector has a higher specificity value, but it would be extremely rare for that to matter.
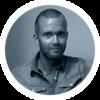
Daniel Fitzhugh
9,715 PointsThanks Steven, that's exactly the answer I was looking for. It's all syntax that I recognise, I just hadn't been able to work it out for myself.
I get your point about not needing to select using a tag name AND an id value.... I just always write CSS with a high level of specificity / scannability / comprehensibility, and it's a practice I intend to replicate in my javascript. This way I can return to the javascript at a later date and understand the structure of the HTML I'm targetting immediately, rather than having to go "hmmm I wonder what this refers to... ?". I hate code structures where you can't immediately work out what's going on, like for example when the teacher gives variables names like "p" and "li".
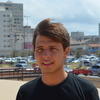
Erwan EL
7,669 PointsSince you already declared the parent you doesn't need to declare it again into the removedListItem variable.
let removedListItem = document.querySelector("li:last-child");
parentList.removeChild(removedListItem);
here you take the parent list then you remove his last child.
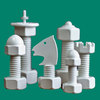
Steven Parker
232,176 PointsThat might not work if "#fullList" was not the first ul on the page. But this would:
let removedListItem = parentList.querySelector("li:last-child"); // start at parent, not document
Erwan EL
7,669 PointsErwan EL
7,669 PointsIf you want to select all li you can use also querySelectorAll('li')