Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial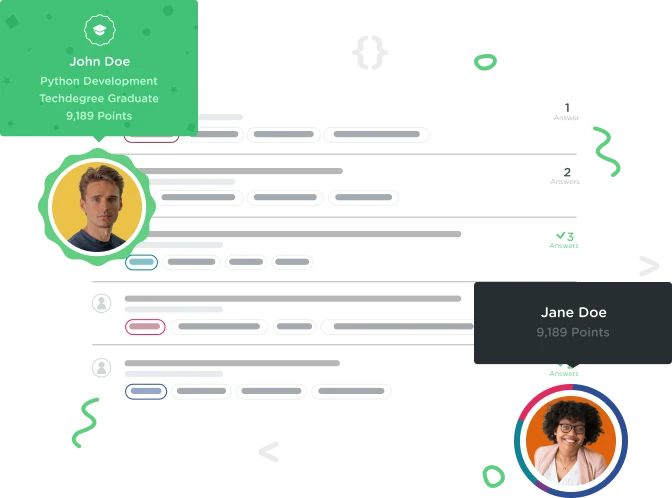

Braden Piper
3,677 PointsHow to Select Only the Elements inside a Specific Tag
In this exercise I'm asked to do this: In the app.js file on line 1, select all links in the nav element and assign them to navigationLinks.
The links in the <nav> element are inside of an <ul> and they all have a <li> tag. I can't simply use:
let navigationLinks = document.getElementsByTagName('li');
because there are other items in the HTML document outside of the <nav> element with the <li> tag.
let navigationLinks = document.getElementsByTagName('nav');
says it is returning only one linkโI don't really understand this, because the <nav> element is not a link. I also tried this:
let navigationLinks = document.querySelector('nav').getElementsByTagName('li');
and it is telling me "the wrong links or elements were selected.
Given the methods demonstrated in the lesson, I can't find a way to do what this is asking. Any help would be appreciated.
Here is the HTML for reference:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
2 Answers
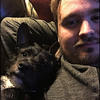
Matthew Long
28,407 PointsYou can select them like you would in CSS. Using descendant selectors.
let navigationLinks = document.querySelectorAll('nav a');
let galleryLinks = document.querySelectorAll('ul#gallery a');
let footerImages = document.querySelectorAll('footer img');
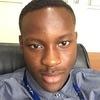
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsThis is one way to go about the challenge Braden:
let navigationLinks = document.querySelectorAll('nav ul li a');

Braden Piper
3,677 PointsCan you explain what the letter "a" refers to? I really don't know much about CSS. I'm doing the beginner Javascript course. Maybe I should switch over and learn a little beginner CSS before continuing?
Braden Piper
3,677 PointsBraden Piper
3,677 PointsCan you explain what the letter "a" refers to? I really don't know much about CSS. I'm doing the beginner Javascript course. Maybe I should switch over and learn a little beginner CSS before continuing?
Matthew Long
28,407 PointsMatthew Long
28,407 Pointsa
refers to an anchor tag. Think if it as a link. In the above, the anchor tag is nested inside the nav tag:There are other elements nested too, but you don't need to get that specific.
Knowing how to select elements in the DOM will help with this portion of JavaScript for sure. I thought that the beginner track had beginner CSS and HTML at the start.
Raviv Fontaine
4,270 PointsRaviv Fontaine
4,270 PointsHi Matthew:
Just wanted to say thanks for this explanation a few months later, as someone else who struggled since the content of this challenge wasn't covered in the prior videos! I appreciate it.