Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial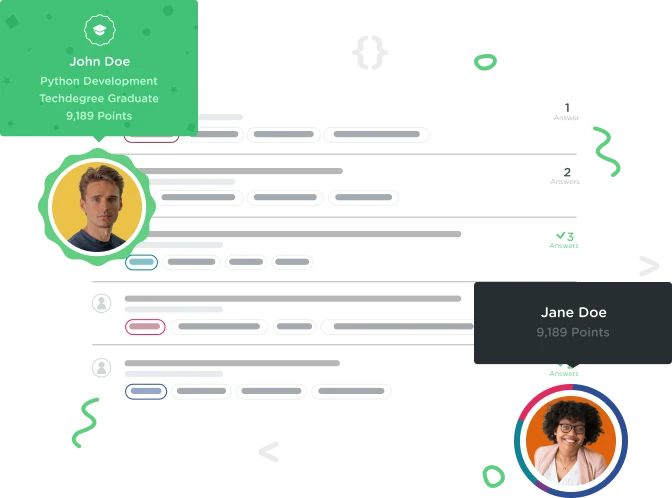

Maie Bauza
653 Pointshow to send data to MySQL with AJAX + jQuery + PHP
this is my contact.html
<!-- form -->
<script type="text/javascript" src="js/form-validation.js"></script>
<form id="contactForm" action="contact_form.php" method="post">
<h3 class="heading">Contact us using this form</h3>
<p> </p>
<fieldset>
<div>
<input name="name" id="name" type="text" class="form-poshytip" title="Enter your full name" />
<label>Name</label>
</div>
<div>
<input name="email" id="email" type="text" class="form-poshytip" title="Enter your email address" />
<label>Email</label>
</div>
<div>
<input name="contact_no" id="contact_no" type="text" class="form-poshytip" title="Enter your Contact Number" />
<label>Contact Number</label></label>
</div>
<div>
<textarea name="comments" id="comments" rows="5" cols="20" class="form-poshytip" title="Enter your comments"></textarea>
</div>
<p><input type="button" value="Send" name="submit" id="submit" /> <span id="error" class="warning">Message</span></p>
</fieldset>
</form>
<p id="sent-form-msg" class="success">Form data sent. Thanks for your comments.We will update you within 24 hours. </p>
<!-- ENDS form -->
this is my form-validation.js
jQuery(document).ready(function($){
// hide messages
$("#error").hide();
$("#sent-form-msg").hide();
// on submit...
$("#contactForm #submit").click(function() {
$("#error").hide();
//required:
//name
var name = $("input#name").val();
if(name == ""){
$("#error").fadeIn().text("Name required.");
$("input#name").focus();
return false;
}
// email
var email = $("input#email").val();
if(email == ""){
$("#error").fadeIn().text("Email required");
$("input#email").focus();
return false;
}
// contact_no
var contact_no = $("input#contact_no").val();
if(contact_no == ""){
$("#error").fadeIn().text("Contact number required");
$("input#contact_no").focus();
return false;
}
// comments
var comments = $("#comments").val();
// data string
var dataString = 'name='+ name
+ '&email=' + email
+ '&contact_no=' + contact_no
+ '&comments=' + comments
// ajax
$.ajax({
type:"POST",
data: dataString,
success: success()
});
});
// on success...
function success(){
$("#sent-form-msg").fadeIn();
$("#contactForm").fadeOut();
}
return false;
});
this is my form.php
<?php
$con=mysqli_connect("localhost","root","rinu","inquiry_box");
// Check connection
if (mysqli_connect_errno()) {
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
// escape variables for security
$name = mysqli_real_escape_string($con, $_POST['name']);
$email = mysqli_real_escape_string($con, $_POST['email']);
$contact_no = mysqli_real_escape_string($con, $_POST['contact_no']);
$comment = mysqli_real_escape_string($con, $_POST['comment']);
$sql="INSERT INTO contact_form (name, email, contact_no, comment)
VALUES ('$name', '$email', '$contact_no', '$comment')";
if (!mysqli_query($con,$sql)) {
die('Error: ' . mysqli_error($con));
}
echo "1 record added";
mysqli_close($con);
?>
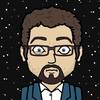
Mitchell Reynolds
3,063 PointsHi Maie, Is there any error messages being displayed? If so, where does it say the error is within your code?
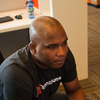
Fabion Stephens
Courses Plus Student 5,893 PointsCan you post the actual form code please?

Ryan Duchene
Courses Plus Student 46,022 PointsEdited your post to fix syntax highlighting.
5 Answers

Grzegorz Doleczek
4,815 PointsHi, you have lot of code in your JavaScript and HTML file, when I search for error, I try to mock some quick/small version of my code to see if everything is OK.
So I created stripded down version of form:
<form action="postprocess.php" method="post" id="contactForm">
<input type="text" name="name"/>
<input type="email" name="email"/>
<input type="text" name="contact_no"/>
<textarea name="comments" id="" cols="30" rows="10"></textarea>
<input type="submit"/>
</form>
And I did the same to JS code:
$('#contactForm').submit(function(){
var self = this;
// All the validation code
$.ajax(
$(this).attr('action'),
{
data: $(self).serialize(),
method: $(self).attr('method'),
success: function(){
// we have positive response.
}
}
);
return false;
});
It is important to notice that:
1) I use submit event on form, and not click event on submit button.
2) Serialization of the form is done by .serialized() , so it is more flexible, and less prone to errors

Grzegorz Doleczek
4,815 PointsCan't find any obvious code errors, beside this one:
$.ajax({
type:"POST",
data: dataString,
success: success()
});
should be like that:
$.ajax({
'type':"POST",
'data': dataString,
'success': success
});
you have to pass name of the function, not the result of it. But back to original problem, check out if your MySQL credetials are ok,

Maie Bauza
653 Pointsthe only thing problem that data does not enter into database it so the problem seems in the javascript which i think ajax please help me

Maie Bauza
653 PointsjQuery(document).ready(function($){
// hide messages
$("#error").hide();
$("#sent-form-msg").hide();
// on submit...
$("#contactForm #submit").click(function() {
$("#error").hide();
//required:
//name
var name = $("input#name").val();
if(name == ""){
$("#error").fadeIn().text("Name required.");
$("input#name").focus();
return false;
}
// email
var email = $("input#email").val();
if(email == ""){
$("#error").fadeIn().text("Email required");
$("input#email").focus();
return false;
}
// contact_no
var contact_no = $("input#contact_no").val();
if(contact_no == ""){
$("#error").fadeIn().text("Contact number required");
$("input#contact_no").focus();
return false;
}
// comments
var comments = $("#comments").val();
// data string
var dataString = 'name='+ name
+ '&email=' + email
+ '&contact_no=' + contact_no
+ '&comments=' + comments
// ajax
$.ajax({
type:"POST",
url: "form.php",
data: dataString,
success: success()
});
});
// on success...
function success(){
$("#sent-form-msg").fadeIn();
$("#contactForm").fadeOut();
}
return false;
});

Maie Bauza
653 Points<!-- form -->
<script type="text/javascript" src="js/form-validation.js"></script>
<form id="contactForm" action="form.php" method="post">
<h3 class="heading">Contact us using this form</h3>
<p> </p>
<fieldset>
<div>
<input name="name" id="name" type="text" class="form-poshytip" title="Enter your full name" />
<label>Name</label>
</div>
<div>
<input name="email" id="email" type="text" class="form-poshytip" title="Enter your email address" />
<label>Email</label>
</div>
<div>
<input name="contact_no" id="contact_no" type="text" class="form-poshytip" title="Enter your Contact Number" />
<label>Contact Number</label></label>
</div>
<div>
<textarea name="comments" id="comments" rows="5" cols="20" class="form-poshytip" title="Enter your comments"></textarea>
</div>
<p><input type="button" value="Send" name="submit" id="submit" /> <span id="error" class="warning">Message</span></p>
</fieldset>
</form>
<p id="sent-form-msg" class="success">Form data sent. Thanks for your comments.We will update you within 24 hours. </p>
<!-- ENDS form -->

Maie Bauza
653 Pointsplease help me i want my form send it to mysql please how should i do
Maie Bauza
653 PointsMaie Bauza
653 Pointsi really dont understand what is the probleme why data does not enter into database (mysql) phpmyadmin please help me Sorry if i send long Message :)