Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial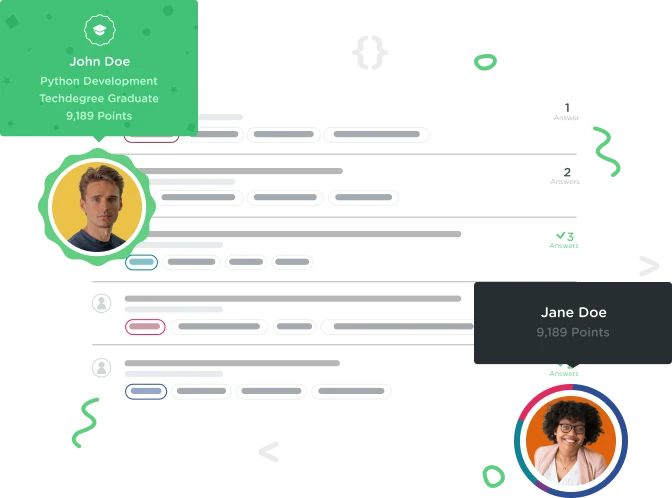

Malcolm Mutambanengwe
4,205 Pointshow to set image using butterknife
I thought I'd get this one but butterknife is a bit confusing. Steve Hunter
import android.os.Bundle;
import android.view.View;
public class MovieActivity extends Activity {
@BindView(R.id.movieTitle) TextView mTitleLabel;
@BindView(R.id.movieImage) ImageView mMovieImage;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_movie);
ButterKnife.bind(this);
// The rest of the code is omitted for brevity :)
}
public void updateDisplay(Movie movie) {
mTitleLabel.setText(movie.getTitle());
mMovieImage.setImageDrawable(movie.getImageId(int mImageId));
}
}
1 Answer
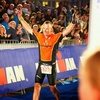
Steve Hunter
57,712 PointsHi Mal,
You've got the first bit spot on - you've correctly identified that you want to call setImageDrawable
on mMovieImage
. Good work.
You've also got the right method for movie
which is getImageId()
- but that doesn't need a parameter; it just returns the image id so leave it as movie.getImageId()
.
What you need to add is a call to getDrawable()
- the question mentions this. The parameter for getDrawable
is the image id that we just wrote. So, getDrawable(movie.getImageId())
would work fine. If you remember from the video, we're getting resources, right? So, we want to chain, using dot notation, the getDrawable()
call on to a getResources()
method. ALL of this is passed into setImageDrawable()
as its parameter. That makes for a long paramater, but it looks like this:
mMovieImage.setImageDrawable(getResources().getDrawable(movie.getImageId()));
I hope that explanation helps and you understand it!
Steve.
Malcolm Mutambanengwe
4,205 PointsMalcolm Mutambanengwe
4,205 PointsI got it master Steve Hunter, the coding force is beginning to like me.
Drawable drawable = getResources().getDrawable(movie.getImageId()); mMovieImage.setImageDrawable(drawable);
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsPerfect!