Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial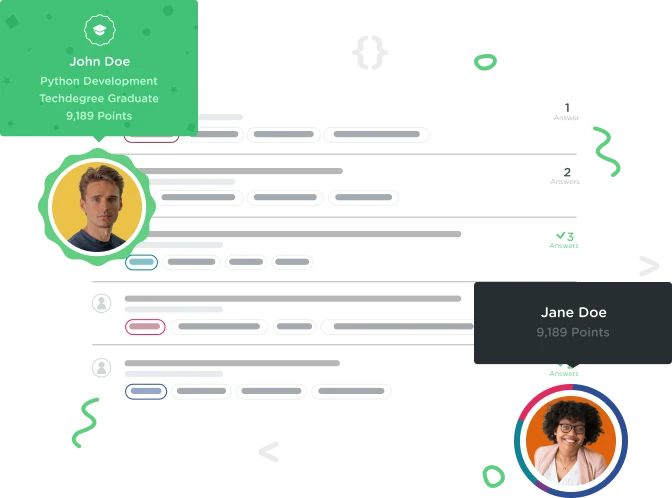

chz
5,407 PointsHow to set up UISearchBar in UICollectionView?
I'm trying to add in the ability to search through my CollectionView. Currently I've added the search bar in storyboard, and I've added UISearchBarDelegate and UISearchDisplayDelegate to my CollectionView.h file which looks like:
#import <UIKit/UIKit.h>
#import <Parse/Parse.h>
#import "Cell.h"
#import "DetailViewController.h"
@interface CollectionViewController : UICollectionViewController <UICollectionViewDelegate, UICollectionViewDataSource, UISearchBarDelegate, UISearchDisplayDelegate> {
NSArray *imageFilesArray;
}
@property (strong, nonatomic) IBOutlet UICollectionView *imagesCollection;
@property (strong, nonatomic) IBOutlet UISearchBar *searchBar;
@property (strong, nonatomic) NSMutableArray *filteredArray;
@end
My CollectionView.m file is as follows:
#import "CollectionViewController.h"
@interface CollectionViewController ()
@end
@implementation CollectionViewController
@synthesize imagesCollection;
@synthesize searchBar;
@synthesize filteredArray;
static NSString * const reuseIdentifier = @"MainCell";
- (void)viewDidLoad {
[super viewDidLoad];
[self queryParseMethod];
self.searchDisplayController.displaysSearchBarInNavigationBar = YES; >
self.filteredArray = [NSMutableArray arrayWithCapacity:[imageFilesArray count]];
}
#pragma mark Content Filtering
-(void)filterContentForSearchText:(NSString*)searchText scope:(NSString*)scope {
[self.filteredArray removeAllObjects];
NSPredicate *predicate = [NSPredicate predicateWithFormat:@"SELF contains %@",searchText];
filteredArray = [NSMutableArray arrayWithArray:[imageFilesArray filteredArrayUsingPredicate:predicate]];
}
From here on the protocol in a table view would be as follows, but I'm not sure how to achieve this same result with a CollectionView.
#pragma mark - UISearchDisplayController Delegate Methods
-(BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchString:(NSString *)searchString {
[self filterContentForSearchText:searchString scope:
[[self.searchDisplayController.searchBar scopeButtonTitles] objectAtIndex:[self.searchDisplayController.searchBar selectedScopeButtonIndex]]];
return YES;
}
-(BOOL)searchDisplayController:(UISearchDisplayController *)controller shouldReloadTableForSearchScope:(NSInteger)searchOption {
[self filterContentForSearchText:self.searchDisplayController.searchBar.text scope:
[[self.searchDisplayController.searchBar scopeButtonTitles] objectAtIndex:searchOption]];
return YES;
}
Does anyone have any ideas of how to implement this in the CollectionVIew?