Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial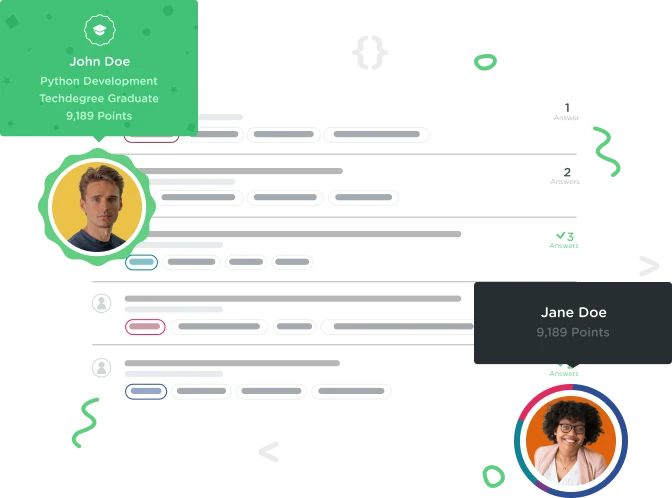
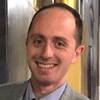
Clifford Gagliardo
Courses Plus Student 15,069 PointsHow to show monster?
I am not sure how to go about showing the monster. I tried to add the monster variable to the print command at the end of the draw_map() function, but that doesn't work.
3 Answers

Stefano Nebiolo
1,991 PointsI got the same problem! If you show it as displayed in the comment I do not have any problem, but if I try to implement a show_position() function taking the draw() function as starting point and adding some if statement like: if cell == monster: output = tile.format("M") It doesn't work properly. It only shows me the door position. Any idea?
Here my code, the clear function is different from kenneth's since I am working on PyCharm
import random
import pyautogui
import sys
# draw grid
# pick random location for player
# pick random location for exit door
# pick random location for monster
# draw player in the grid
# take input for movement
# move player, unless invalid move (past edges of grid)
# check for win/loss
# clear screen and redraw screen
CELLS = [(0, 0), (1, 0), (2, 0), (3, 0), (4, 0),
(0, 1), (1, 1), (2, 1), (3, 1), (4, 1),
(0, 2), (1, 2), (2, 2), (3, 2), (4, 2),
(0, 3), (1, 3), (2, 3), (3, 3), (4, 3),
(0, 4), (1, 4), (2, 4), (3, 4), (4, 4),
]
# function to clean the screen
def clear_screen():
# os.system('cls' if os.name == 'nt' else 'clear')
pyautogui.hotkey('ctrl', 'l') # no command but ctrl
# get the location of the player, of the door and of the monster
def get_locations():
return random.sample(CELLS, 3)
# move the player according to the move input. player stands for player position
def move_player(player, move):
x, y = player
if move == "LEFT":
x -= 1
if move == "RIGHT":
x += 1
if move == "UP":
y -= 1
if move == "DOWN":
y += 1
return x, y
# check whether the input move can be accepted or not
def get_moves(player):
moves = ["LEFT", "RIGHT", "UP", "DOWN"]
x, y = player
if y == 0:
moves.remove("UP")
if y == 4:
moves.remove("DOWN")
if x == 0:
moves.remove("LEFT")
if x == 4:
moves.remove("RIGHT")
return moves
# draw the map showing the player position
def draw_map(player):
print(" _"*5)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < 4:
line_end = ""
if cell == player:
output = tile.format("X")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X")
else:
output = tile.format("_|")
print(output, end=line_end)
def play_again():
print("Do you want to play again? Y/N?")
again = input("> ").upper()
if again == "Y":
game_loop()
elif again == "N":
print("Bye Bye!")
sys.exit()
else:
print("Sorry, I didn't get what you want to do. Tell me again")
play_again()
def set_win(player, door, monster):
# set win
if player == door:
print("You found the door! You Escaped!")
show_position(player, door, monster)
play_again()
# set loss
elif player == monster:
print("Oh no! The monster ate you!")
print("The door was in {}!".format(door))
show_position(player, door, monster)
play_again()
def show_position(player, door, monster):
print(" _" * 5)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < 4:
line_end = ""
if cell == player:
output = tile.format("X")
if cell == monster:
output = tile.format("M")
if cell == door:
output = tile.format("D")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X")
if cell == monster:
output = tile.format("M")
if cell == door:
output = tile.format("D")
else:
output = tile.format("_|")
print(output, end=line_end)
def game_loop():
monster, door, player = get_locations()
print(door)
print(monster)
while True:
draw_map(player)
valid_moves = get_moves(player)
print("You are currently in room {}".format(player))
print("You can move {}".format(", ".join(valid_moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
elif move in valid_moves:
player = move_player(player, move)
else:
input("\n ** Walls are hard! Don't run into them ** \n") #input instead of print so we have to insert return or whatever to keep playing. In this way our clear function is nt deleting our Careful message
set_win(player, door, monster)
clear_screen()
clear_screen()
print("Welcome to the Dungeon!")
input("Press return to start")
clear_screen()
game_loop()

David Fisher
3,375 PointsI had the same problem. It took a little trial and error but I finally figured this out. Adding an elif for the monster is correct, but the monster variable needs too be added to the draw_map function in both it's definition, and it's inclusion within the game_loop function. This should work to show the door as well if desired. It should looks like this in both places:
def draw_map(player, monster):
print(" _"*5)
tile = "|{}"
for cell in CELLS:
x, y = cell
if x < 4:
line_end = ""
if cell == player:
output = tile.format("X")
elif cell == monster:
output = tile.format("M")
else:
output = tile.format("_")
else:
line_end = "\n"
if cell == player:
output = tile.format("X|")
elif cell == monster:
output = tile.format("M|")
else:
output = tile.format("_|")
print(output, end=line_end)
while playing:
clear_screen()
draw_map(player, monster)
valid_moves = get_moves(player)
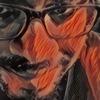
Kevin Akpomuje
1,357 PointsI have got it to show the monster like this:
draw_map(player)
valid_moves = get_moves(player)
# fill with player position
print("You're currently in room {}".format(player))
# fill with available moves
print("You can move {}".format(", ".join(valid_moves)))
# is monster & door set??
print("Monster is in {}".format(monster))
print("Door is in {}".format(door))
print("Enter QUIT to end the game.")
```
Flore W
4,744 PointsFlore W
4,744 PointsWhat error do you get?
I had the same issue at some point (it drew the map with only the Door but not the Monster) because I used several "if" instead of one "if" and other "elif". Try replacing the show_position function using elif and see if it works.