Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial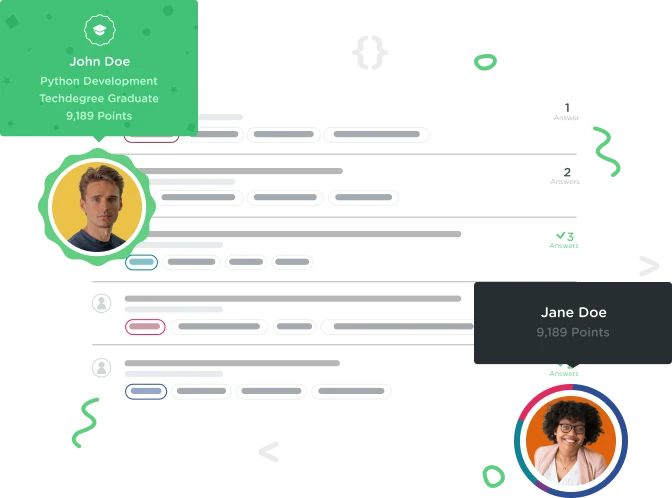

Misho Milicevic
5,597 PointsHow to show multiples of a UIImageView on screen?
I'm trying to create a game that has an effect similar to firing bullets. I'm using a UIImageView and trying to create an array that copies the image and displays it as many times as the fire button is pushed. My main issue is the first image fired will disappear if another is fired, meaning there's only one image being used - no copies. This is what I have so far that has been working:
-(void)placeProjectile {
projectile.hidden = NO;
// This places the projectile near the object it is fired from. The object here is part of a different method
projectile.center = CGPointMake(object.center.x - 5, object.center.y + 10);
}
-(void)projectileMoving {
projectile.center = CGPointMake(projectile.center.x + 5, projectile.center.y + 20);
//This gives the impression of the projectile moving with the road after it lands on it
if (CGRectIntersectsRect(projectile.frame, hitRoad.frame)) {
projectile.center = CGPointMake(projectile.center.x - 7, road.center.y);
}
}
-(IBAction) fire:(id)sender {
[self placeProjectile]; [self projectileMoving]; }
Given the above code (which works), how would I go about creating an array that allows more than one projectile to be fired at a time? I'm leaving out all the various attempts I made at it. PLEASE HELP! I've been stuck on this for weeks :(
1 Answer
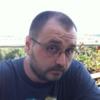
Robert Bojor
Courses Plus Student 29,439 PointsHi Misho,
Have you tried creating a new instance of the projectile every time the user taps? The code you have now uses one instance, as soon as you reset it the old one will disappear - pretty much what's going on in your code by what you were saying.
By creating new instances of the projectile and adding them to the view will ensure that the old ones will continue to exist. And right about now is where you are going to run into trouble again. You will need to control all the instances and move them accordingly...
I would suggest using Sprite Kit, since it sounds like a game, plus you won't need to micromanage all the instances of objects you create. There is a really nice and easy to follow project about Sprite Kit here on Treehouse - check the Library.
Misho Milicevic
5,597 PointsMisho Milicevic
5,597 PointsHi Robert,
Thanks for your reply. Thank you again for your advice. I will attempt to create a new instance of the projectile as you suggested - mainly for practice and gaining a better understanding of how to structure my coding. SpriteKit as you mentioned is definitely the route I want to take. I started the SpriteKit tutorial already and I'm almost done. Thank you again for your help, I sincerely appreciate it.