Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial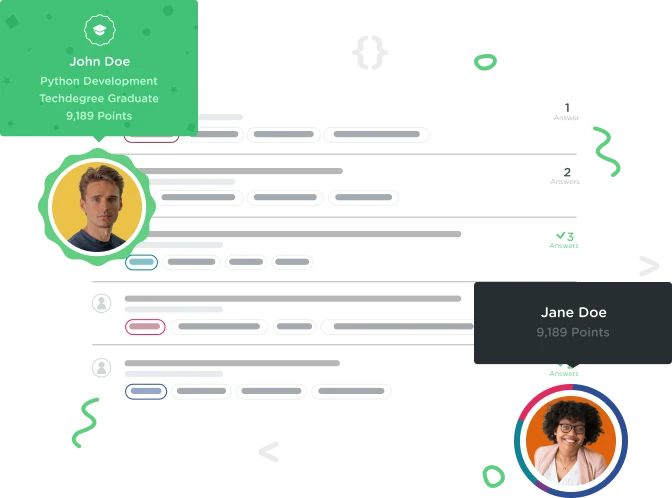
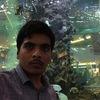
Nagaraju Banoth
Courses Plus Student 5,617 PointsHow to solve Challenge Task 3 of 3 in Error Handling in Swift 3?
Challenge Task 3 of 3
Perfect! We're almost done. Rather than a generic catch clause, let's pattern match on the specific errors so that we can log better error messages. In this task, change the catch statement to include specific error cases. Like before you can just log an error message of your choosing.
enum ParserError: Error {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.EmptyDictionary
}
guard let key = data["somekey"] else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
do {
let parser = try Parser(data: data)
try parser.parse()
} catch ParserError.EmptyDictionary {
print("error")
}
catch
ParserError.InvalidKey {
print("errorr")
}
Raed Alahmari
12,966 PointsRaed Alahmari
12,966 Points