Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial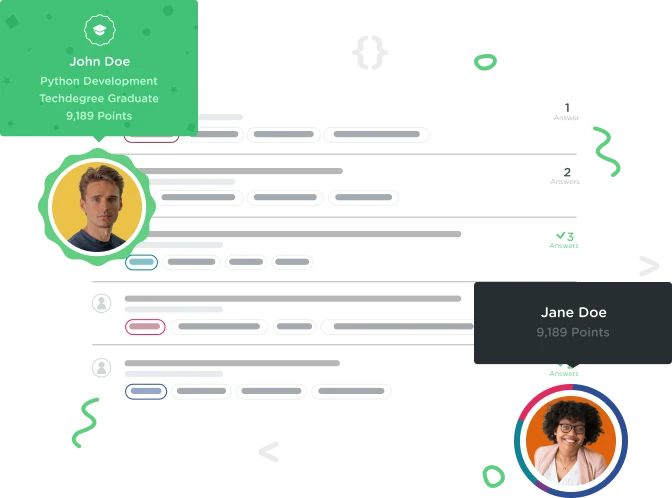
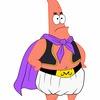
<noob />
17,062 PointsHow to solve the multiple name problem?
Hello, this is the current snapshot: https://w.trhou.se/nqz913rr3d
I have solved the problem when that dealt with if the user is not exists thanks to one of steven's answers. However i just want to be 100% sure. I used a flag to determine wheter or not a user is in our database and i did those check only after the loop ends. We do it because we need to finish to loop through the all students? I tried to add an else clause and even if i typed a student that is in the database i got the output from the else clause.
I'm having some problems figure out how to handle the multiple name issue. Can i get any pointers?
8 Answers

KRIS NIKOLAISEN
54,968 PointsSince you set found = true in your loop if a name matches, but still loop through all names, your code will always print the last student if a student has been found. So you will need a means of identifying which student was found.
Instead of a boolean you could make found an array of found students. Then outside the loop check the length of the array to determine if there are students to print. You'd have to deal with the same name searched more than once. But this would allow you to print multiple positive search results as well as two or more students with the same name.
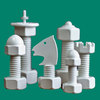
Steven Parker
230,274 PointsThe suggestion of the boolean test was intended to go after the search (inner) loop, but still inside the main loop. And that test was just for showing the "not found" message.
The printing of the student record can be done in the same place as where the boolean is set to true
, and that way it will run for each student found. And don't forget to set it back to false
before beginning another search.
Kris's suggestion of building an array of found students is another viable strategy, but it would require another loop after the main one to display the array.
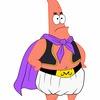
<noob />
17,062 Pointsi tried to implement what u suggested and it doesn't working as expected this is the current snapshot: https://w.trhou.se/eg63ptww9i
i set the flag to false after the loop ends and when even if i serach an existing name i got the message - user not found.
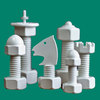
Steven Parker
230,274 Points if(!found) // you still need to perform this test
{ // to ony show message when NOT found
print("user not found");
} //
found = false; // then reset before next search
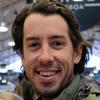
Tom Madsen
Front End Web Development Techdegree Graduate 19,030 PointsHere is my solution, for what it's worth...
var message = ''
var student;
var search;
var correct = []
var searchHistory = []
function print(message) {
let div = document.getElementById('output');
div.innerHTML = message
}
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>'
report += '<p>Track: ' + student.track + '</p>'
report += '<p>Achievements: ' + student.achievements + '</p>'
report += '<p>Points: ' + student.points + '</p>'
return report
}
while (true) {
search = prompt('Please enter the student name you require the records of, otherwise type \'quit\' to exit the program')
if (search.toLowerCase() === 'quit' || search === null) {
break
}
searchHistory.push(search)
for (let i = 0; i < students.length; i++) {
student = students[i]
if (student.name === search) {
message += getStudentReport(student)
print(message)
correct.unshift(student.name)
}
if (correct[0] === correct[1]){
correct.shift()
}
}
if (correct.length < searchHistory.length) {
alert('There are no students by that name, sorry!')
searchHistory.pop()
}
}
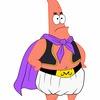
<noob />
17,062 Pointscan u explain what u did in this lines:
if (correct[0] === correct[1]){
correct.shift()
}
}
if (correct.length < searchHistory.length) {
alert('There are no students by that name, sorry!')
searchHistory.pop()
}
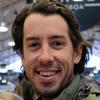
Tom Madsen
Front End Web Development Techdegree Graduate 19,030 PointsThe correct array was added primarily to later measure its length against the length of the searchHistory array.
So, for each time through the loop of students' names, if the search matched student.name, correct.length added 1. If there were 2 instances of the same name, correct length would still only be incremented by 1 because of the correct.shift().
The alert message would only be called after each prompt if , after iterating through the loop, there were no matching strings.
The searchHistory.pop() removed the incorrect array item so that array.length wouldn't keep getting bigger casuing the alert message to continually pop up when it shouldn't.
Side note, I haven't tested this with 3 or more of the same student.name value. It probably wouldn't work... :)

KRIS NIKOLAISEN
54,968 PointsIf you want to see my modification to your code here it is. I'm sure it could use some work but this is what I was talking about.
Note: There are three idans. If you search for one all three are returned.
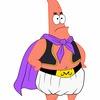
<noob />
17,062 PointsI liked ur solutions. but how exactly the includes() method works? because the way its working currently is ur first check if
if(student.name === searchName)
and if so then u check if it returns false, which means teh found array don't have the specified searchName in him. but how u still get the three idans to get into that found array if after the first idan the second check evluate to true?
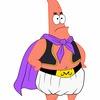
<noob />
17,062 Pointsi need to put it before or after the while map? Steven Parker
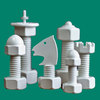
Steven Parker
230,274 PointsIf you mean my comment from Thursday, 6/20 at 4:38pm, it replaces lines 50-52 — inside the "while", after the "for".

KRIS NIKOLAISEN
54,968 PointsIn my code found is an array of student objects
if(student.name === searchName)
matches the name then if true a check is done to see if the student object exists in the array
if (found.includes(student) === false)
In this case the entire student object not just the name is verified. That way when the loop proceeds and finds the second idan by name, it is not a second item in the array as an object, so the second idan is added.
If you were to search for idan a second time the objects would be found so they would not be added. That way they wouldn't double up.
w3schools on includes()