Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial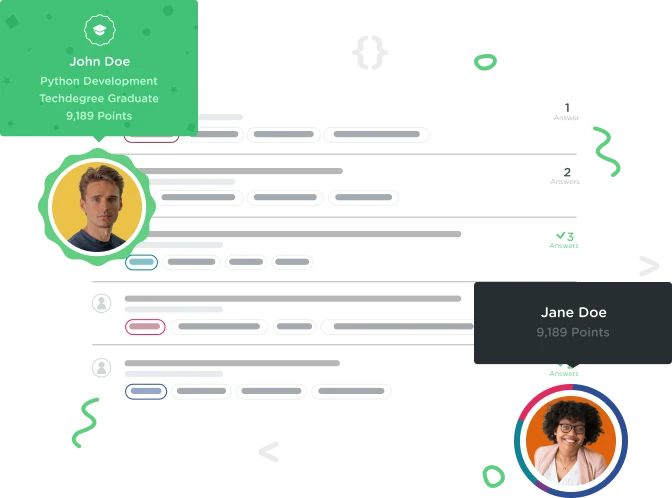

Saleh Bubishate
1,613 PointsHow to solve this challenge?
How to solve this task,,,
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car : Vehicle {
var numberOfSeats : Int
override init(withDoors doors: Int, andWheels wheels: Int, numberOfSeats : Int = 4 ) {
super.init( numberOfDoors = doors, numberOfWheels = wheels)
self.numberOfSeats = numberOfSeats
}
}
let someCar = Car(doors: 2, andWheels wheels: 3, numberOfSeats: 4)
1 Answer
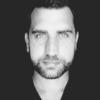
Jhoan Arango
14,575 PointsHello,
There are number of rules when it comes to initializing a class that inherits from a super class.
One of those rules is :
When initializing your class, you must first give values to the class's properties, then you call the super's init. Also, if the class that you are defining is inheriting all of the super class's properties and is not defining any properties of its own, you must call super.init before you can modify any of the properties.
So in this challenge we must do it as follows:
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int = 4
init(numbersOfSeats: Int) {
// We first give values to "numberOfSeats
self.numberOfSeats = numbersOfSeats
// Then we call super init
super.init(withDoors: 2, andWheels: 4)
}
}
let someCar = Car(numbersOfSeats: 2)
Since Car is defining its own property numberOfSeats, then we must give a value to that property before calling super.init.
Hope this helps.
Also remember that if this answer was of any help, don't forget to help others by selecting this answer as best answer, or if you need a better explanation please let me know.
Good luck
Saleh Bubishate
1,613 PointsSaleh Bubishate
1,613 Pointsthanks a lot,, in the subclass,, once I entered init,,,, xcode suggests to change it to override init,,, do I need to do that or just to type init?
Saleh Bubishate
1,613 PointsSaleh Bubishate
1,613 Pointsone more thing,,, why did you entered 2 for "withDoors" argument and 4 for "andWheels" argument? I tried to type super.init( withDoors : withDoors, andWheels : andWheels) but there was error
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsHello,
You only use "override" when the subclass is going to create its own implementation of the inherited initializer of the super class. In this case, you do not need to override it since you are creating an initializer that is different from the super class.
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsPerhaps this would be a better solution
Remember, you first have to initialized the store properties of "Car" before calling super.init.
For more information you can read "The Swift Programming Language" from Apple.
Click "here"