Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial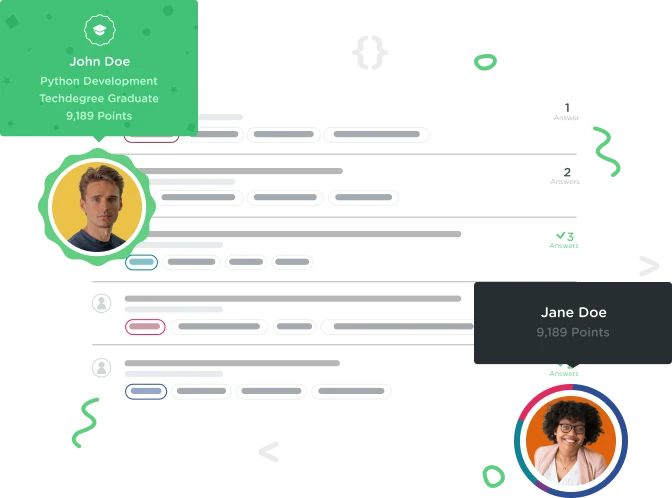

Rajasekhar Reddy Nalla
7,894 PointsHow to solve this challenge
How to solve this challenge
package com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.ui.ModelMap;
@Controller
public class ContactController {
@RequestMapping("/")
public String home() {
return "index";
}
@RequestMapping("/contact")
public String contact(ModelMap modelMap) {
Contact c = new Contact();
modelMap.put("contact",c);
return "contact_detail";
}
}
package com.teamtreehouse.contactmgr.model;
public class Contact {
private int id;
private String firstName;
private String lastName;
private String email;
public Contact() {}
public Contact(int id, String firstName, String lastName, String email) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public int getId() {
return id;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getEmail() {
return email;
}
public void setId(int id) {
this.id = id;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setEmail(String email) {
this.email = email;
}
}
3 Answers
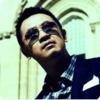
xinhuang79
Courses Plus Student 918 Pointschange your contact to
@RequestMapping("/contact/{id}") //added the id parameter public String contact(@PathVariable int id, ModelMap modelMap) { //added the @PathVariable... Contact c = new Contact(); c.setId(id) //added assign id modelMap.put("contact",c); return "contact_detail"; }

Dean Vollebregt
24,583 PointsThanks xinhuang79, that worked great
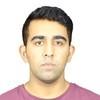
Awais Jamil
7,539 Pointspackage com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.ui.ModelMap;
@Controller public class ContactController {
@RequestMapping("/")
public String home() {
return "index";
}
@RequestMapping("/contact/{id}")
public String contact(@PathVariable int id, ModelMap modelMap) {
Contact c = new Contact();
c.setId(id);
modelMap.put("contact",c);
return "contact_detail";
}
}
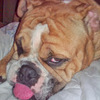
Shane Robinson
7,324 PointsCould you tell us specifically what part you are stuck on? That would help us know how to guide you.

Rajasekhar Reddy Nalla
7,894 PointsThe question for the above challenge is: In ContactController.java, update the contact method so that it captures an int id in the URI, such as "/contact/8" or "/contact/723". Then, use this captured value as the id for a new Contact object you construct and add to the ModelMap. Don't worry about the firstName, lastName, or email.
Please give me a solution for this.
Xayaseth Boudsady
21,951 PointsXayaseth Boudsady
21,951 PointsSee Comments