Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial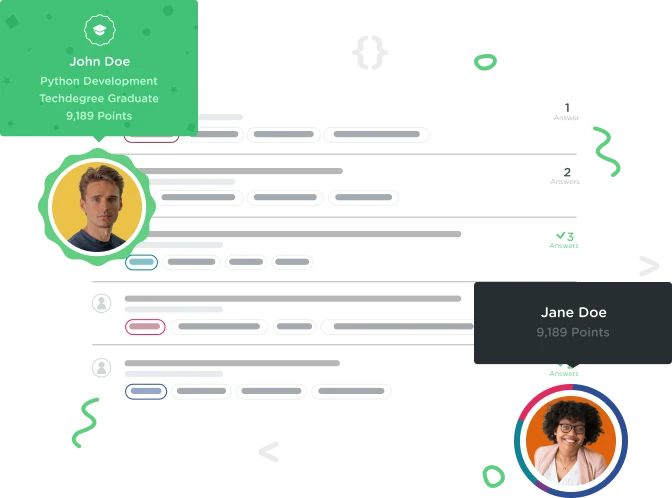

john peter
Courses Plus Student 1,215 Pointshow to solve this im a bit confused by the question
What does the question mean?
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class FunFactsActivity extends Activity {
private TextView factTextView;
private Button showFactButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign them the Views from the layout file
TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton;
}
}
2 Answers

Mahmoud Amin
Courses Plus Student 6,269 Points```import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class FunFactsActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign them the Views from the layout file
TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
}
}```

Moira Lawrie-Martyn
8,073 PointsSo in this question, they're asking you to grab the two View variables and cast them into Java so that the program behind the layout can recognise the layout.
In this situation they're wanting you to take:
private TextView factTextView;
private Button showFactButton;
and tell Android where to find them in the layout, which you've almost got:
// Declare our View variables and assign them the Views from the layout file
TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton;
You'd want to change "factLabel" to "factTextView" so it matches the variable declared. You'd also want to take out the "TextView" part before it because it's already been declared.
With the button, it'd be the same. First off take out the "Button" part because it's already been declared, then add in the assignment which would be showFactButton = (Button) findViewById(R.id.showFactButton); which takes the showFactButton variable, tells Java it's looking for a button and where that button is in the layout.
Hope this helps :)