Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial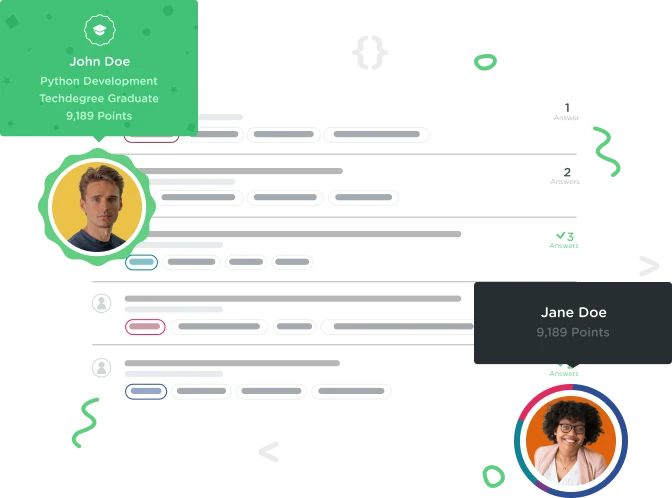
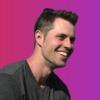
Michael Sothan
8,048 Pointshow to sort a list built inside of a child class?
I don't understand why the self.slots list will not sort.
So, if my list was
my_list = SortedInventory()
then I would have a list named "my_list". So, assuming there's no sort code,
my_list.add_item("cookie", "banana")
would give me a list like [cookie, banana] right? But, with the sort code, from my understanding, since python will automatically inherit the "list.sort" function, then the
my_list.add_item("cookie", "banana")
method call should also run the "self.slots.sort()" code which (if we ran "print(my_list)") should output: [banana, cookie], no?
What am I missing? Do I need to put something in the "()" of "sort"? Or should the "list.sort()" code be placed inside of a separate method in the SortedInventory class?
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.append(item)
self.slots.sort()
3 Answers

Josh Keenan
19,652 PointsYou're really close, look at the parent class, add_item
already adds your item to the slots, you are doing it twice with your SortedInventory
version of it too.
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.sort()
Your code is adding the item, then adding it again, then sorting it, which is causing it to fail the challenge. You got this!
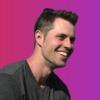
Michael Sothan
8,048 PointsGot it. Thanks Josh! I now see that I was misunderstanding the use of super(). I thought you had to re-write the code block from the parent class's method under your super() call. In this case:
self.slots.append(item)
I thought that seemed Highly redundant. Now I see that with the super() call in the child class you can use the code block from the parent class without rewriting anything! Thanks

Brian Davis
1,628 PointsThat helps a lot. Is it just me, or is there being a lot left out in the lectures?
Michael Sothan
8,048 PointsMichael Sothan
8,048 PointsThanks Josh. The second question in the challenge asked us to override the add_item method using super() which is why there is the extra add_item() in the child class. I passed that part, so I can't imagine that is the issue.
I tried it again using the following code (without the super() ):
But to no avail. Any idea?
Josh Keenan
19,652 PointsJosh Keenan
19,652 PointsYour problem is the extra append you have as I said in that comment,
You are adding it in the original method,
super()
is doing that for you, you need to remove it in the child class, readd the super, my code is what I used to pass it