Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial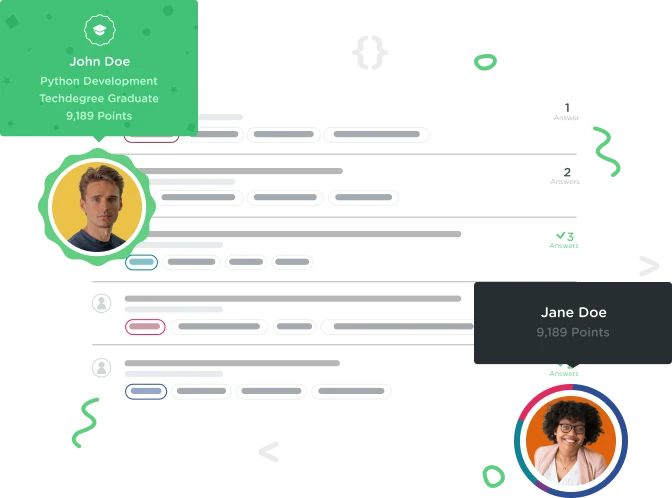

Allison Seim
1,738 Pointshow to split a string without a separator
how to match an alphabet of a string with the key of a dictionary
def morse_code(word):
morse_dict = {
'a': 'dot-dash',
'b': 'dash-dot-dot-dot',
'c': 'dash-dot-dash-dot',
'd': 'dash-dot-dot',
'e': 'dot',
'f': 'dot-dot-dash-dot',
'g': 'dash-dash-dot',
'h': 'dot-dot-dot-dot',
'i': 'dot-dot',
'j': 'dot-dash-dash-dash',
'k': 'dash-dot-dash',
'l': 'dot-dash-dot-dot',
'm': 'dash-dash',
'n': 'dash-dot',
'o': 'dash-dash-dash',
'p': 'dot-dash-dash-dot',
'q': 'dash-dash-dot-dash',
'r': 'dot-dash-dot',
's': 'dot-dot-dot',
't': 'dash',
'u': 'dot-dot-dash',
'v': 'dot-dot-dot-dash',
'w': 'dot-dash-dash',
'y': 'dash-dot-dash-dash',
'z': 'dash-dash-dot-dot'
}
a = list(word)
for i in a:
if i == morse_dict.keys():
return (morse_dict.values())
# enter your code below
morse_code("govern")
1 Answer
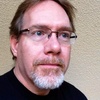
Chris Freeman
Treehouse Moderator 68,457 PointsHey Allison Seim.
Strings can certainly be split
, but not with an empty separator:
>>> "abcde".split('')
Traceback (most recent call last):
File “<stdin>”, line 1, in <module>
ValueError: empty separator
The Pythonic way is to treat the string as a container, like a list
. A str
has an __iter__
method that will yield one character at a time.
So, you can do:
>>> list("abcde")
[‘a’, ‘b’, ‘c’, ‘d’, ‘e’]
>>> for char in "abcde":
... print(char)
...
a
b
c
d
e
Your list(word)
is perfect.
Since the whole alphabet is in morse_dict
you don’t need the if
, simply look up the character morse_dict[i]
. The append this result to a list that can be later joined into a string. (Be sure to use a hyphen when joining)
Post back if you need more help. Good luck!!!
Lening Li
689 PointsLening Li
689 PointsYou can just do:
morse_dict.get(alphabet, '')