Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial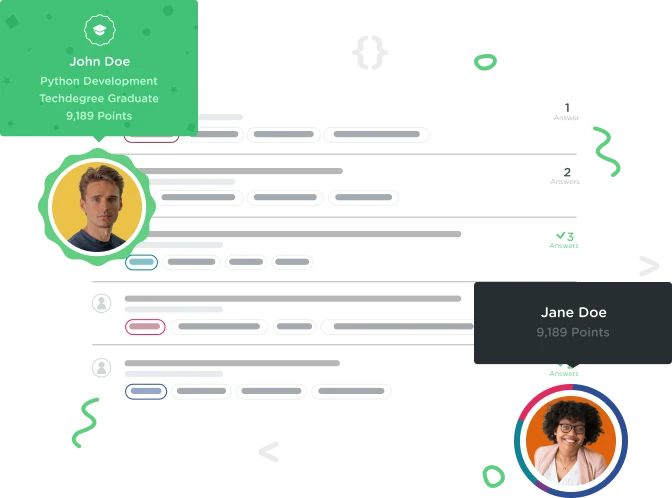
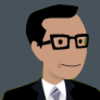
armanb21
848 PointsHow to stop a task running in Objective -C.
Hey guys,
I was implementing the pull to refresh in my app but I'm not sure how I can stop the instance from happening. I'm stuck in a infinite loading loop.
The code I used for the pull to refresh is here:
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
UIRefreshControl *refreshControl = [[UIRefreshControl alloc] init];
[refreshControl addTarget:self action:@selector(refresh:) forControlEvents:UIControlEventValueChanged];
[self.colorsTable addSubview:refreshControl];
[self retrieveFromParse];
}
- (void)refresh:(id)sender
{
}
So how can I get the loop that keeps going on and on in a circle to stop after 6 seconds?
Thanks for your help,
Arman
1 Answer
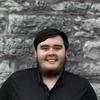
Michael Hulet
47,912 PointsThe way you have it set up, the refresh:
method is called every time the user attempts to pull to refresh, to handle the actual refreshing. If you want to only stop the spinning animation after 6 seconds and not do anything else, an implementation might look like this:
-(void)refresh:(id)sender{
if([(NSObject *)sender isKindOfClass:[UIRefreshControl class]]){
UIRefreshControl *spinner = (UIRefreshControl *)sender;
//There's probably a better way to do this with NSTimer, but that solution would be a little complicated for the Treehouse Forum, so I'll just use C's sleep function here
sleep(6);
//On its own, the UIRefreshControl class doesn't know when you're done refreshing, and therefore, won't know when to stop its animation, until you tell it to
[spinner endRefreshing];
}
}
armanb21
848 Pointsarmanb21
848 PointsThanks.