Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial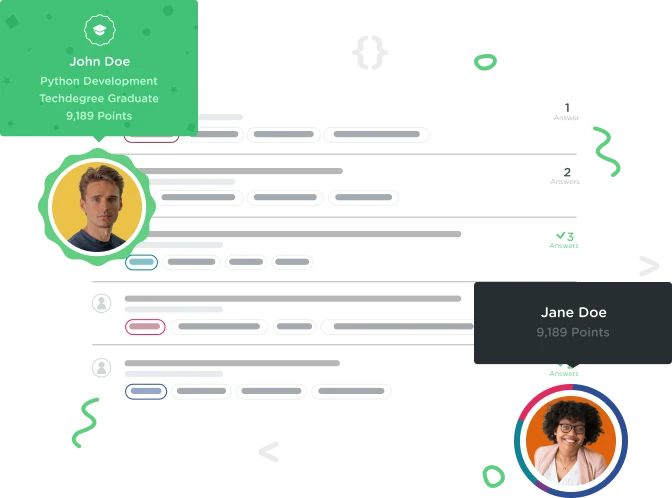

Abdi Ahmed
7,151 Pointshow to stop JavaScript window reloading when each time button is on a page.
Hi guys,
just trying to fix the an issue with my code in JS, basically I'm creating a simple hot or cold app that generates a random number and the user has to guess the number. The user is allowed as many guesses as they need to guess the correct number. My problem is that each time the button is clicked to enter a number, the page reloads and generates a new random number. I had it working fine, but mysteriously this bug has come about. Here is the code for JS - I think the problem is coming from the processGuess method in the controller object but I'm not sure how to fix it:
var globalValue;
var view = {
displayMessage: function(msg, count) {
var messageArea = document.getElementById("feedback");
messageArea.innerHTML = msg;
}
};
function generateRandomNumber(){
var randomNumber = Math.floor(Math.random() * 101);
return randomNumber;
}
var generatedNumber = generateRandomNumber();
console.log(generatedNumber);
var controller = {
guesses: 0,
processGuess: function(guess) {
var diff = Math.abs(generatedNumber - guess);
if (guess <=0 || guess >=101) {
alert("Oops, please enter a number between 1 & 100");
} else if(diff >= 50){
view.displayMessage("Ice cold");
} else if (diff >= 30 && diff < 50){
view.displayMessage("Cold");
} else if (diff >= 20 && diff < 30){
view.displayMessage("Warm");
} else if (diff >= 10 && diff < 20){
view.displayMessage("Hot");
} else if (diff >= 1 && diff < 10){
view.displayMessage("Very hot");
}
this.guesses++;
return null;
}
}
function init() {
/*--- Display information modal box ---*/
$(".what").click(function(){
$(".overlay").fadeIn(1000);
// $(".overlay").show();
});
/*--- Hide information modal box ---*/
$("a.close").click(function(){
$(".overlay").fadeOut(1000);
// $(".overlay").hide();
});
var fireButton = document.getElementById("guessButton");
fireButton.onclick = handleFireButton;
var guessInput = document.getElementById("userGuess");
guessInput.onkeypress = handleKeyPress;
}
function handleFireButton() {
var guessInput = document.getElementById("userGuess");
// var guess = parseInt(guessInput.value);
var globalValue = parseInt(guessInput.value);
controller.processGuess(globalValue);
guessInput.value = "";
}
function handleKeyPress(e) {
var fireButton = document.getElementById("guessButton");
if (e.keyCode === 13) {
fireButton.click();
return false;
}
}
window.onload = init;
And here's the HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hot || Cold</title>
<!-- Meta Tags -->
<meta charset="utf-8"/>
<!-- Stylesheets -->
<link rel="stylesheet" href="styles/reset.css">
<link href='http://fonts.googleapis.com/css?family=Lato:400,700,900,900italic' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="styles/style.css"/>
<!-- JavaScript -->
</head>
<body>
<header> <!--Header -->
<!-- Top Navigation -->
<nav>
<ul class="clearfix">
<li><a class="what" href="#">What ?</a></li>
<li><a class="new" href="#">+ New Game</a></li>
</ul>
</nav>
<!-- Modal Information Box -->
<div class="overlay" id="modal">
<div class="content">
<h3>What do I do?</h3>
<div>
<p>This is a Hot or Cold Number Guessing Game. The game goes like this: </p>
<ul>
<li>1. I pick a <strong>random secret number</strong> between 1 to 100 and keep it hidden.</li>
<li>2. You need to <strong>guess</strong> until you can find the hidden secret number.</li>
<li>3. You will <strong>get feedback</strong> on how close ("hot") or far ("cold") your guess is.</li>
</ul>
<p>So, Are you ready?</p>
<a class="close" href="#">Got It!</a>
</div>
</div>
</div>
<!-- logo text -->
<h1>HOT or COLD</h1>
</header>
<section class="game"> <!-- Guessing Section -->
<h2 id="feedback">Make your Guess!</h2>
<form>
<input type="text" name="userGuess" id="userGuess" class="text" maxlength="3" autocomplete="off" placeholder="Enter your Guess" />
<input type="submit" id="guessButton" class="button" name="submit" value="Guess"/>
</form>
<p>Guess #<span id="count">0</span>!</p>
<ul id="guessList" class="guessBox clearfix">
</ul>
</section>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
<script type="text/javascript" src="js/app1.js"></script>
</body>
</html>
Many thanks
2 Answers

Petros Sordinas
16,181 PointsHi Abdi,
The problem is that you are using a input of type submit for your "Guess" button. The HTML thinks you want to send the form data to a server for processing so it refreshes.
Either change the guessbutton to a button element or change your button click handler to this:
document.getElementById("guessButton").addEventListener("click", function(event){
event.preventDefault();
handleFireButton();
});
The event.preventDefault() function will prevent the browser from performing the default action on the submit button, i.e. it will prevent it from submitting the form and will proceed to the next line, calling your function.
Hope this makes sense.

Abdi Ahmed
7,151 PointsThank you Petros! That makes a lot of sense and it has solved my issue. Thank you for taking the time to help me I really appreciate!
Thanks

Petros Sordinas
16,181 PointsGlad to help! :)