Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial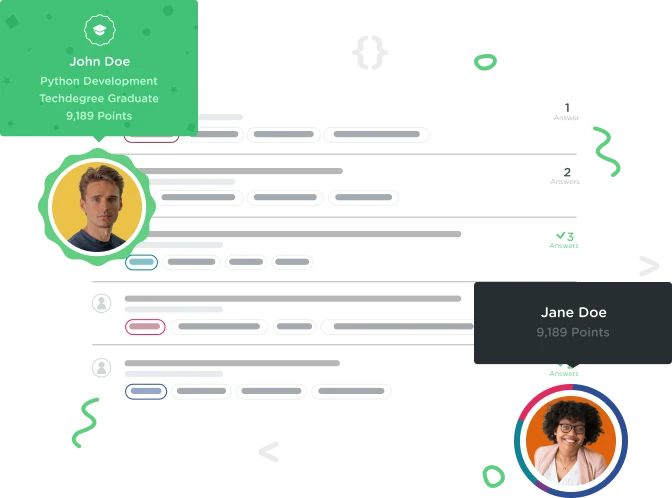

Mahfuzur Rahman
3,204 Pointshow to store var_dump boolean value into a variable
I solved the problem some how. But I don't remember how exactly I did it.
<?php $a=10; $b=20; $isIdentical; var_dump($a===$b); isIdentical=var_dump; echo isIdentical;
?>
2 Answers

Corey Cramer
9,453 Pointsvar_dump is not the right tool for the job you are intending, it's mostly (from personal and anecdotal experience) used for visually debugging your code as it outputs the value of what you are calling it on to the browser or console depending on how you are calling your script. Similar to stuffing echo statements in your code in various places to make sure values are what you intend them to be.
A more appropriate solution would be an if/else statement
<?php
$a = 10;
$b = 20;
if ($a === $b) {
$isIdentical = true;
} else {
$isIdentical = false;
}
echo $isIdentical;
?>
If you are looking for a more terse way of doing it you can use a simple comparison.
<?php
$a = 10;
$b = 20;
$isIdentical = ($a === $b);
echo $isIdentical;
?>
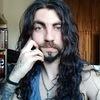
Emil Pesaxov
3,684 PointsYou forgot the dollar sign of 'isIdentical' variable.
So, you can do something like: $c = var_dump($a + $b); echo $c; // echoes int(20);