Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial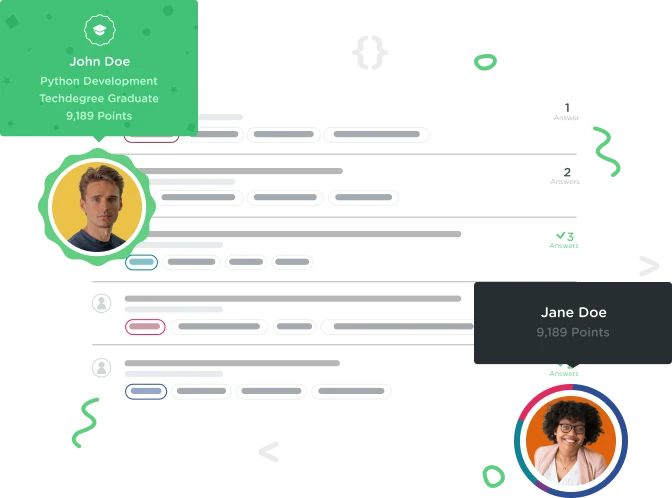
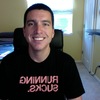
Stephen Printup
UX Design Techdegree Student 45,252 PointsHow to submit a custom object to express with or without jade / pug?
js
let routineExerciseArray = []
window.onload = function () {
$('#workoutName').on('change', function () {
document.getElementById('workoutNameOut').innerHTML = "<hr/>" + "Name: " + $('#workoutName').val()
})
$('#workoutSets').on('change', function () {
document.getElementById('workoutSetsOut').innerHTML = "Sets: " + $('#workoutSets').val()
})
$('#workoutMeta').on('change', function () {
document.getElementById('workoutMetaOut').innerHTML = "Meta: " + $('#workoutMeta').val()
})
$('input[type="checkbox"]').on('change', function() {
if (this.checked == true) {
routineExerciseArray.push(this.id)
} else {
var indexToRemove = routineExerciseArray.indexOf(this.id)
routineExerciseArray.splice(indexToRemove,1)
}
displayWorkout();
});
}
pug
h4.exercises Exercise List
p Choose exercises and name the workout
form(method='POST' action='/buildWorkout')
div.form-group
input.form-control(type='text' id='workoutName' placeholder= 'Workout Name' name='workoutName')
input.form-control(type='text' id='workoutSets' placeholder= 'Workout Sets' name='workoutSets')
input.form-control(type='text' id='workoutMeta' placeholder= 'Workout Meta Info' name='workoutMeta')
br
ul
each val in exerciseArray
input(type="checkbox", id=val.exerciseName)
| #{val.exerciseName} | #{val.exerciseDuration}
br
div(id="workoutNameOut")
div(id="workoutSetsOut")
div(id="workoutMetaOut")
div.form-group(id="workoutDisplay" name='workoutExercises')
button.btn.btn-primary(type='submit') Save Workout
I've dynamically added a checkbox that displays with documents from mongo. When the checkboxes are selected, they build an array in the js file. I'm trying to store this object (name, sets, meta and exercises) into mongo, but don't know how to either 1.)access the request object from the js file or 2.) pass the created array of items to the jade template to submit with the form. or 3.) any other way to pass the created object to express to store in mongo.
Thank you Huston Hedinger
1 Answer
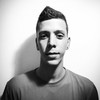
Jesus Mendoza
23,288 PointsHey Stephen,
You just need to use the body-parser package.
var express = require('express');
var bodyParser = require('body-parser');
var path = require('path');
var app = express();
app.use(bodyParser.urlencoded({ extended: true });
app.use(bodyParser.json());
app.get('/', function(req, res) {
res.sendFile(path.join(__dirname, '/index.html'));
}
// Now you need to submit your form to this url (you can change it).
app.post('/', function(req, res) {
/* Inside your callback func you will have access to the
req.body object with all the properties submitted from your html
in this example (req.body.firstName) but you can change it or add more. */
console.log(req.body);
});
app.listen(5000, function(err) {
if (err) throw err;
console.log('Server is up and running!');
});
Now, in your form you set the action to the url you defined in your application, then when you submit your form, you will have access using req.body and the name set in your form (in this case req.body.firstName).
<form method="POST" action="/">
<input type="text" name="firstName" id="firstName">
<input type="submit">
</form>
Stephen Printup
UX Design Techdegree Student 45,252 PointsStephen Printup
UX Design Techdegree Student 45,252 PointsThanks for your answer Jesus Mendoza. I have the first three properties displaying in the req.body (name, set, meta), but the 4th (an dynamically created array of exercises) is not passed and is undefined. Since the array is created using checkboxes (with each checked exercise being added to the array), the array changes based on user input. This array of custom selected exercises is what is not passed in the req.body. I have a div with name='workoutExercises', but I don't know how to make it submit the user generated content when the submit button is clicked. Does that make sense? Having a div with the right name doesn't make that div hold the array. How do I make the div hold the array of selected exercises? I have the array created, but I don't understand how to make jade read the array (in scripts.js).
Jesus Mendoza
23,288 PointsJesus Mendoza
23,288 PointsStephen Printup You can add the same name property to all the checkboxes and it will be sent as an array if you select more than 1, else it will send a string
Stephen Printup
UX Design Techdegree Student 45,252 PointsStephen Printup
UX Design Techdegree Student 45,252 PointsYes!! And, just fyi I had to set the value attribute to the desired return value and it works perfect now. Thank you Jesus Mendoza!!
Jesus Mendoza
23,288 PointsJesus Mendoza
23,288 PointsYeah, values can be change though. Be careful with that!