Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial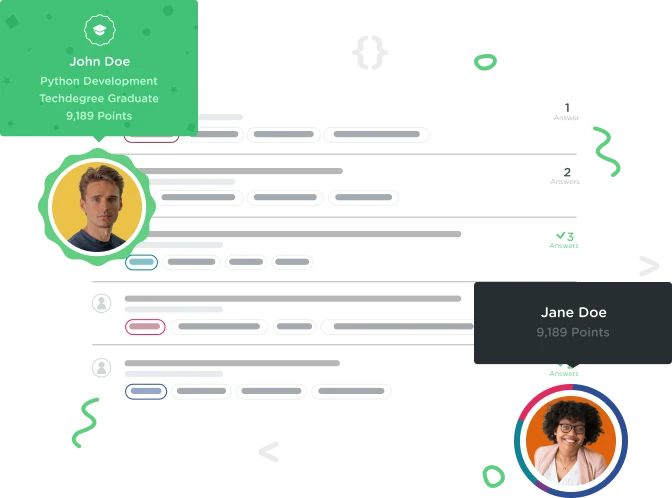

Darrel Lyons
2,991 PointsHow to submit on select
I'm quite new to Javascript and i'm trying to find out how i submit on select and update the database.
I have this so far:
<form method="post">
<select name='myfield' onchange='form.submit()'>
<option>Select Weapon</option>
<option>AK47</option></select>
<noscript><input type="submit" value="Submit"></noscript>
</form>
But i have no idea how to update the database from here.
1 Answer

Heidi Brebels
23,239 PointsHello,
Personally, I use JQuery to do this:
Give your form and select an id:
<form method="post" id="myform">
<select name='myfield' id="myfield">
Then at the bottom of your page, before the end of your body:
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<script>
$('#myfield').bind('change', function () {
//post
$("#myform").submit();
});
</script>
Your page will be reloaded and since your form uses the method POST, you can get the value of the select from the $_POST["myfield"] using PHP. (this "myfield" is the name of the name attribute, not the id, the id is for JQuery, PHP uses name):
<?php
$selectedvalue = $_POST["myfield"];
?>
You can further use PHP to update your Database with this value. Best is to use PDO, there's a course here. Something like this:
<?php
$selectedvalue = $_POST["myfield"];
//you'll probably need this for a specific user or something else?
$userid = 1;
updateDatabase($selectedvalue, $userid);
//preferable in another .php file:
function updateDatabase($selectedweapon, $userid) {
require("_inc/database.inc.php");
try {
$query = "UPDATE T_User
SET myweapon = :selectedweapon
WHERE userid = :userid";
$results = $db_mnet->prepare($query);
$results->bindParam(':selectedweapon',$selectedweapon,PDO::PARAM_STR);
$results->bindParam(':userid',$userid,PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
exit;
}
}
?>
You could fill in the selected value again on reload in your select too:
<form method="post" id="myform">
<select name='myfield' id="myfield">
<option>Select Weapon</option>
<option <?php if (isset($selectedvalue) && ($selectedvalue == "AK47")) { echo "selected"; } ?>>AK47</option>
</select>
</form>
Darrel Lyons
2,991 PointsDarrel Lyons
2,991 PointsI've done it , thank you very much!!! :)