Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial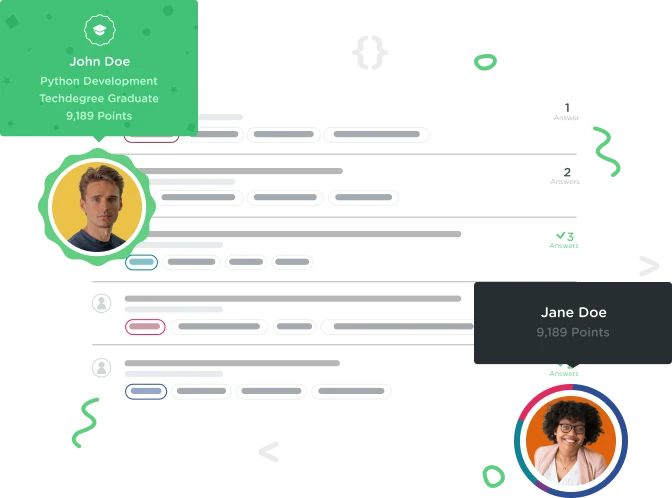
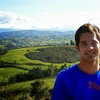
Dhruv Ghulati
1,582 PointsHow to take comma separated inputs for list, challenge part 2?
First part of challenge solved via:
print(','.join(shopping_list))
For the second part, would I include error handling via:
if not ',' in input('>'):, print('You have an invalid input'), else shopping_list.append(new_item)?
3 Answers
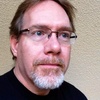
Chris Freeman
Treehouse Moderator 68,423 PointsYour error handling, as written, requires that the input must have a comma. Does this mean you wish to not allow adding items one at a time?
Instead of checking for a comma on the input, you can simply split()
the input into a list. The code below captures input into new_items
then adds them to the shopping_list
. Note the use of extend()
instead of append()
since split()
returns a list:
new_items = input('>')
shopping_list.extend(new_item.split(','))
If the input is a single item, split
simply returns a list consisting of that one item. Caution: split
only removes the splitting character. If you split on comma and the user includes spaces then the spaces will be part of the item string. It will take more code to handle both cases of commas and commas+spaces. Perhaps removing all spaces before splitting or parsing the input with a intermediate Python regular expression
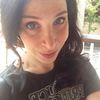
Marni Kostman
7,558 Pointschris freeman Here is the code I just came up with. It may have some spacing issues but it only uses the functions we have learned up to this point in Kenneth's videos.
shopping_list = [] #could also use list() function
print("What should we pick up at the store?")
print("Enter 'DONE' to stop adding items.")
while True: #true is never a reason to stop...will go on forever
new_item=input("> ")
if new_item == "DONE":
break #breaks the loop
new_item=new_item.split(", ")
for item in new_item:
shopping_list.append(item)
print("Added! List has {} items.".format(len(shopping_list)))
continue #continues the loop/starts over
print("Here's your list:")
for item in shopping_list: #don't have to call them "items" - can call them anything - all elements of container, when we get to last item in list, loop stops
print(item)
I originally just added the line with split but, when I did that, it was counting the whole entry as 1 item. When I added the extra "for" loop, the "Added!" line worked and accurately counted items.
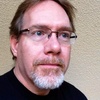
Chris Freeman
Treehouse Moderator 68,423 PointsLooks Good! Three critiques::
- The
continue
statement is not required at the end of awhile
block. Continue is the default action at the end of the code block - You're correct on using "item" over "items" for the iterating variable name since it refers to a singular 'thing' from the reference sequence
shopping_list
-
new_item
might be better named asnew_items
oritems_list
to indicate it is a sequence. The statement then becomes more readable asfor item in new_items: ....
- If user enters a blank item, the empty item will be added to the list. You may wish to add a check for this case and skip adding the blank item
Keep up the good work!
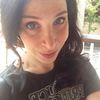
Marni Kostman
7,558 PointsThanks, chris freeman...will definitely update with your suggestions!