Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial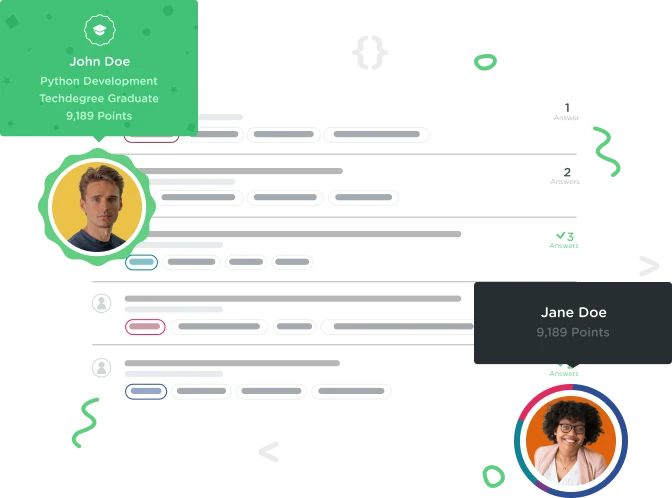

vikas pal
11,563 Pointshow to test it inside the script?
Please see the teachers note of this video and then tell me how to test it from inside the script and then how it run.
1 Answer
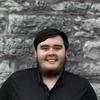
Michael Hulet
47,913 PointsThis is something you'll really only want to do if you're writing a module that is intended to just be import
ed into other modules, and doesn't really provide any functionality on its own when you run it directly (other than running the doctests). For example, let's say I have this file:
def double(a):
"""Returns its input multiplied by 2
>>> double(3)
6
"""
return a * 2
This file only has 1 function, and doesn't do anything other than provide that function when its included. It'd be useful if when it was run on its own, however, if it ran its doctests. You could make it do that by changing its contents to this:
def double(a):
"""Returns its input multiplied by 2
>>> double(3)
6
"""
return a * 2
if __name__ == "__main__": # Check to see if the file is being run by itself
import doctest # Make the code support for doctests available
doctest.testmod() # Tell Python to run all the doctests for this file
Now if we run python multiply.py
, it'll run all the doctests inside of multiply.py
.
On a slightly less useful side note, doctest.testmod
supports setting the m
default argument to something other than __main__
, so if you wanted to test a module inside a different module, you could do that, like this:
import math # Make the math module available
import doctest # Make code support for doctests available
doctest.testmod(m=math) # Run all the tests in the math module