Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial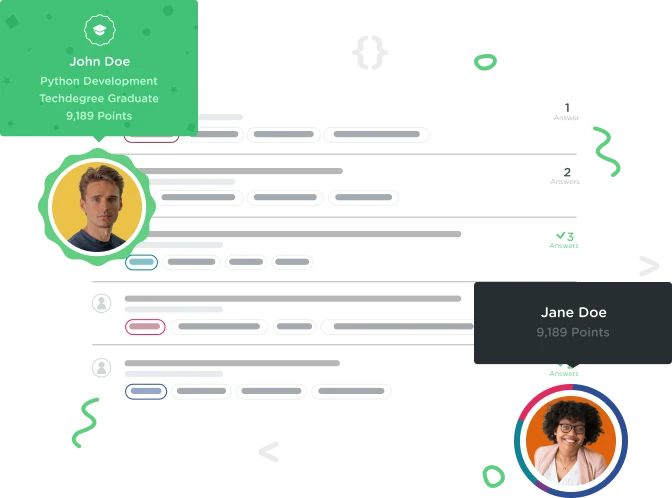

Joseph Lander
Full Stack JavaScript Techdegree Graduate 27,765 PointsHow to test your code for the `drop() method` section?
At this stage, we don't have access to the playToken()
method and we don't have any code for when the "ArrowDown" key is pressed.
I didn't see a way to test if my drop()
method was working. I don't have a target object to test. I don't fully grasp the SVG code to understand the heights I would get.
I had got this far...
drop(target, reset?) {
this.dropped = true;
$(this).animate({
top: // pseudo - full height minus height of full spaces
}, 750, 'easeOutBounce');
}
Q: Is there a way to test this method at this stage or do you write code that should be right, then fine-tune it once you have the actionable method, playToken()
?
I am trying to play along but often I can't see a way to test my code as we progress, which is tough.
2 Answers

Kyle Nel
4,157 PointsHey, it looks like you asked this a while ago but I figured I'd answer it anyway for anyone else who comes looking.
I tested this by adding it to the handleKeydown()
method without passing anything through. The idea is that I'll just manually set the target in my drop()
method while testing to a fixed value. This is useful for testing the transition speed and seeing the animation when you click the down arrow.
My handleKeydown()
method:
handleKeydown(e) {
if (this.ready) {
if (e.key === 'ArrowLeft') {
this.activePlayer.activeToken.moveLeft();
} else if (e.key === 'ArrowRight') {
this.activePlayer.activeToken.moveRight(this.board.columns);
} else if (e.key === 'ArrowDown') {
// notice I'm not passing the property "reset" and I'm manually defining the target for testing
const target = {top: 380}; // manipulated token top in inspector to get this value
this.activePlayer.activeToken.drop();
}
}
}
Then I just use that fake target attribute in my drop()
method:
drop(target, reset) {
const top = {top: (target.y * target.diameter)}; //not used during testing
this.dropped = true;
$(this.htmlToken).animate(target, 500, 'easeOutBounce', reset);
}
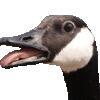
wildgoosestudent
11,274 PointsI love/need to test my code so posting here in case anyone else feels the same!
In Game.js
handleKeydown(e) {
if (this.ready) {
if (e.key == 'ArrowDown') {
// Don't need to pass in any params at this stage to the drop method
this.activePlayer.activeToken.drop()
} else if (e.key == 'ArrowRight') {
this.activePlayer.activeToken.moveRight(this.board.columns)
} else if (e.key == 'ArrowLeft') {
this.activePlayer.activeToken.moveLeft()
}
}
}
In Token.js
drop(target, reset) {
this.dropped = true;
$(this.htmlToken).animate ({
// 76 is the pixel size (target.diameter) and 5 puts it to the bottom
// Can play around with the animate features in here
top: 76 * 5,
duration: 'slow'
})
}