Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial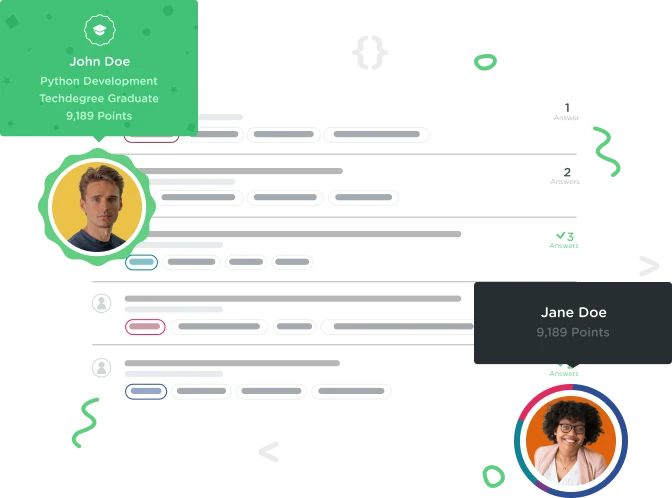

Darin Thompson
5,305 PointsHow to troubleshoot challenges?
I am in the java course and there are some challenges that aren't that simple. And I don't know how to troubleshoot. A lot of code is running in the background and output isn't showing up in the virtual terminal. All you get sometimes is some obscure Bummer! message that seems cryptic. So, what are some tips, if any, for getting input and feedback to help through some of the challenges?
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
for(char tileInTiles : tiles.toCharArray()) {
if (letter == tileInTiles) {
letter++;
}
}
return letter;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
3 Answers
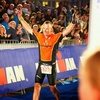
Steve Hunter
57,712 PointsHi Darin,
If you have syntax errors, clicking the preview button will give you the compiler error - this will help you fix your syntax. If the challenge is failing and if there's no errors in preview, your code is syntactically correct but the expected output isn't matching the tests of the challenge. That's when the 'bummer' error message might help you out.
Steve.
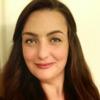
Jennifer Nordell
Treehouse TeacherI agree with Steve Hunter on these points. The most valuable error is a compiler error which will show you a syntax error. I can offer a few additional hints.
If you're getting a compiler error and it shows an error on line 20, but you can't find anything wrong with line 20, start looking upwards in your code. 95% of the time the error is before there.
If you get a "Communication error" when running the challenge, resist the urge to click anything besides the X to close the error. Wait about 30 seconds and then run "Check work" again. If you still get the communication error, run this in a workspace or on your local system with some sort of logging. There's a very real possibility that you've inadvertantly created an infinite loop which will be obvious when you start getting line after line of prints running.
If you get a "Task 1 is no longer passing", it's most likely a syntax error. These challenges are pretty good about showing compiler errors, but sometimes there are exceptions to the rule. Run the code in a workspace and make sure it's compiling.
If you get a very vague "Bummer!" but your code compiles and runs just fine check very carefully the requirements of the challenge. Do all your variable names, function names match to the letter? Did you add new variables when it didn't ask you to? When it's checking output, it must match to the letter. This includes capitalization, spelling, punctuation, and even spacing! In every case it's a great idea to not do anything not explicitly stated by the challenge. Even if functional, it can cause the challenge to fail.
If you think you're overthinking it, you're likely correct.
Hope some of these help!
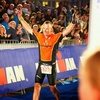
Steve Hunter
57,712 Points

Darin Thompson
5,305 PointsThank for the responses. I was just hoping there was a jshell type thing to get something to work that I know will work and then piece the challenge together. I appreciate the help!