Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial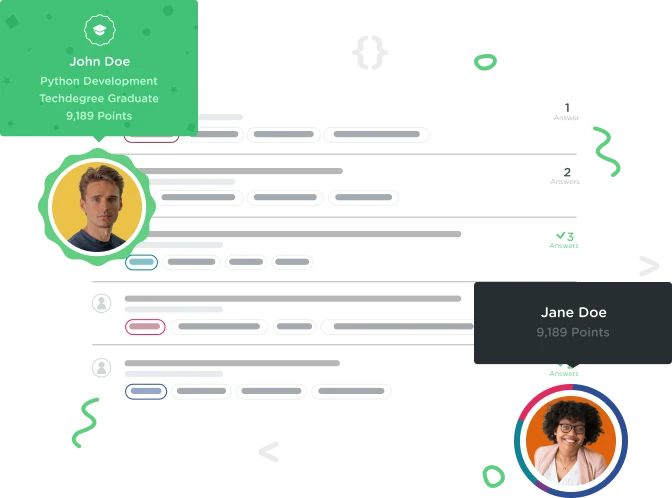

DJreya Boyd
7,268 PointsHow to turn off indent errors
Step 1
Ask the user for their name and the year they were born.
name = input("What is your name?") yearborn = input("What year were you born in") yearnow = 2017
Step 2
Calculate and print the year they'll turn 25, 50, 75, and 100.
year25 = yearborn + 25 year50 = yearborn + 50 year75 = yearborn + 75 year100 = yearborn + 100 print("You'll be 25 in the year{}, 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year25,year50, year75, year100))
Step 3
If they're already past any of these ages, skip them.
if yearnow >= year25: print("You'll be 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year50, year75, year200)) elif yearnow >= year50: print("You'll be 75 in the year {}, 100 in the year {}.".format(year75, year100)) elif yearnow >= year75: print("you'll be 100 in the year {}.".format(year100)) elif yearnow >= year100: print("") else: print("You'll be 25 in the year{}, 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year25,year50, year75, year100))
I keep getting issues for indent errors, where do I learn what gets indented and what doesn't? Also why is it considered an error, I don't like all of my code to start on the same indent, it makes it harder to read.
2 Answers
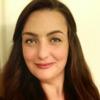
Jennifer Nordell
Treehouse TeacherHi there! You cannot "turn off" indentation errors in Python. Indentation is how Python knows to group together certain statements in a block. It's actually part of the syntax of the language. You can view some documentation on this here.
Furthermore, if when indenting and mixing indentation with tabs and indenting with spaces inside the same .py
file you may receive a "Tab Error". I found a small blog article on that here.
To reiterate, these things are part of the Python language. Just as Python expects tabs to indicate the start of a block, Swift, C#, and Java all expect curly braces to indicate the start and stop of a block. You cannot remove the requirement for indentation any more than you can remove the requirement for curly braces in the other three languages.
Hope this helps!
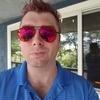
Everett Hubbard
11,446 PointsI am sure that by now you have moved on from this but for anyone else coming across this, there a few options to help.
First, occasionally when working with functions, you may need to move around before completing or closing the function. This can lead to the next line being rolled up into the previous function and even though the indents look correct, the system doesn't see it that way. During this instance, you will want to include the keyword pass at the end of the empty function to close it.
As mentioned by Jennifer Nordell, legitimate indent errors will generally occur when mixing spaces with tabs. There are a few things you could do to minimize these instances.
When you are finished with a block and need to start a new line, use Shift + Enter instead of Enter. Enter may assume the new line is a part of the block and include an indent.
The other extremely beneficial option I utilize to ensure my code stays aligned is within the Workspace itself. If you go to the top menu and select View, make sure that Indent Guides has been turned on.
One last side note. When sharing code, I found that if you add four spaces to the beginning of each line, if creates an easy to read text box that keeps most of the formatting intact. I'm not sure why that's not in the Markdown Cheatsheet but it is definitely a useful formatting option.
name = input("What is your name?")
yearborn = input("What year were you born in")
yearnow = 2017
yearborn = int(yearborn)
year25 = yearborn + 25
year50 = yearborn + 50
year75 = yearborn + 75
year100 = yearborn + 100
print("You'll be 25 in the year{}, 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year25,year50, year75, year100))
if yearnow >= year25:
print("You'll be 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year50, year75, year100))
elif yearnow >= year50:
print("You'll be 75 in the year {}, 100 in the year {}.".format(year75, year100))
elif yearnow >= year75:
print("you'll be 100 in the year {}.".format(year100))
elif yearnow >= year100:
print("")
else:
print("You'll be 25 in the year{}, 50 in the year {}, 75 in the year {}, 100 in the year {}.".format(year25,year50, year75,
year100))
I did find a typo with one of the 100s being 200. And I added a line to your code: yearborn = int(yearborn)