Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial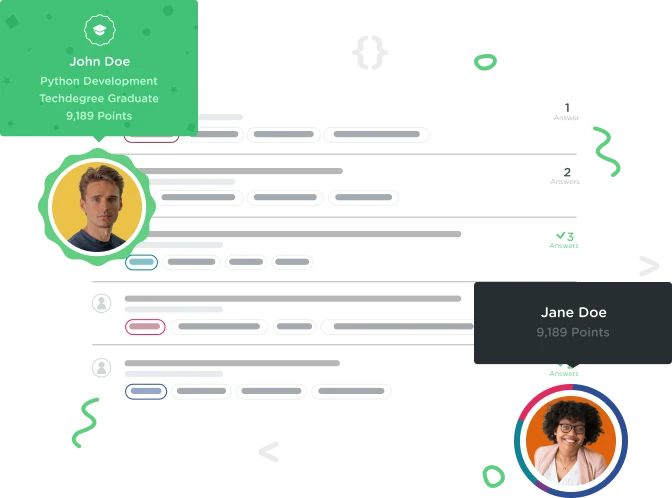

Dimitris Karatsoulas
5,788 PointsHow to turn the yellow background input back to white.
I noticed that all inputs turned yellow click after click. I thought that It'd be a cool and more proffesional feature to make it so that after a click a yellow input would turn back to white. I gave it a try but with no success. You can see my code below. What is really strange and suprising is that even if I use a && condition it seems it's not working according to the JavaScript standards. Can you possibly tell me what's wrong? *Please note I have used the lightblue color for comparison reasons. When I was using RGB (255, 255, 255) for white color I could see no changes in my preview.
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (e.target.tagName === "INPUT"){
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
section.addEventListener('click', (e) => {
if (e.target.tagName === "INPUT" && e.target.style.backgroundColor === 'rgb(255, 255, 0)'){
e.target.style.backgroundColor = 'rgb(0, 255, 255)';
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>JavaScript is an exciting language that you can use to power web servers, create desktop programs, and even control robots. But JavaScript got its start in the browser way back in 1995.</p>
<hr>
<p>Things to Learn</p>
<ul>
<li>Item One: <input type="text"></li>
<li>Item Two: <input type="text"></li>
<li>Item Three: <input type="text"></li>
<li>Item Four: <input type="text"></li>
</ul>
<button>Save</button>
</section>
<script src="app.js"></script>
</body>
</html>
10 Answers

KRIS NIKOLAISEN
54,971 PointsYou could loop through the input elements and clear the background color. In doing so you would no longer need to check the background color with an if condition.
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (e.target.tagName === "INPUT"){
clearInputs()
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
function clearInputs() {
let inputs = document.getElementsByTagName('input');
for (let item of inputs) {
item.style.backgroundColor = '' ;
}
}

KRIS NIKOLAISEN
54,971 PointsYour code sets the color to yellow, then checks if its yellow and if so sets it to blue. So the result is always blue. Try something like this:
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (e.target.tagName === "INPUT"){
if (e.target.style.backgroundColor == '') {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
} else {
e.target.style.backgroundColor = '';
}
}
});

Dimitris Karatsoulas
5,788 PointsThank you, I should had figure this out. What about changing the color when we click on another input though? I mean when we click on the next input the previous one turns back to white so that at any given time we have at most just one colored input.

KRIS NIKOLAISEN
54,971 Pointse.target is just the individual element clicked so it should be the only one affected

Dimitris Karatsoulas
5,788 PointsThanks, so we should another method to achieve that effect?

Dimitris Karatsoulas
5,788 PointsThat's a completely new loop for me! The for...of I mean. Thank you so much for showing this to me!

KRIS NIKOLAISEN
54,971 PointsI originally tried for .. in and had errors. Then I read this stack overflow which says to never use for..in to iterate an HTMLCollection. So new to me too.

Dimitris Karatsoulas
5,788 PointsKnowing how to search for the right answers through Stack Overflow is a must have skill for any developer!

Dimitris Karatsoulas
5,788 PointsMay I also ask why even though for both HTML collections (section and input) we use the getElementsByTagName for section you use [index] but not for input?

Dimitris Karatsoulas
5,788 PointsI think I figured this out. For the section collection we need to work on the 1st element of the HTML collection even if it's the only one. For the input HTML collection though we need to run through all of the elements of the collection using the loop.

KRIS NIKOLAISEN
54,971 PointsAdding the index of 0 specifies the first element (only) in the collection. For inputs
we want to retrieve all elements so no index.

Dimitris Karatsoulas
5,788 PointsThanks again, you're most helpful. I did figure this out by myself just after I posted the question.