Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial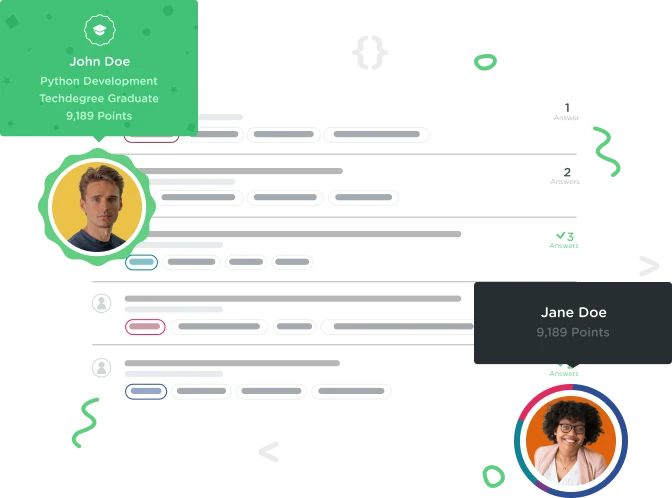
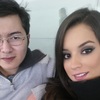
Luis Carlos Rúa Sánchez
Courses Plus Student 1,754 PointsHow to update a listview real time
How to update the listview when some changes are made in the server. I found a lot of approaches but anything had work for me. What is the best practice to do this. Suppose you have a MySQL server and you get some data the first time the app start. But the user make some change in a register. Then i want that to be reflected in the listview (suppose a price had changed or a product is unavailable now)
2 Answers
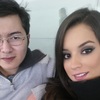
Luis Carlos Rúa Sánchez
Courses Plus Student 1,754 PointsPush notifications is not very advanced topic. And yes that's the best way

Sebastian Röder
13,878 PointsYou say correctly that currently the data is pulled from the server only once -- when the app is started for the first time. This is because the code that gets data from the server and updates the view is called from the onCreate()
method. Example from my code:
// MainListActivity.java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar);
if (isNetworkAvailable()) {
try {
URL feedURL = new URL(FEED_URL);
new FetchBlogDataTask().execute(feedURL);
} catch (MalformedURLException e) {
logException(e);
}
}
}
If you want to get the latest data every time the Activity is started, you can put the relevant code (everything inside the if-block) into the onResume()
method instead. You can read about the lifecycle of Android Activities at the Android Developer website.
This could look something like this:
// MainListActivity.java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar) findViewById(R.id.progressBar);
}
@Override
protected void onResume() {
super.onResume();
if (isNetworkAvailable()) {
try {
URL feedURL = new URL(FEED_URL);
new FetchBlogDataTask().execute(feedURL);
} catch (MalformedURLException e) {
logException(e);
}
}
}
You will learn more about this topics in the Build a Self-Destructing Message Android App course.
If you really want the changes on the server to be displayed in realtime on the app, I guess you must use Push Notifications for that, but this is a really advanced topic. You could also try to implement a Pull to refresh gesture for the list view.