Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial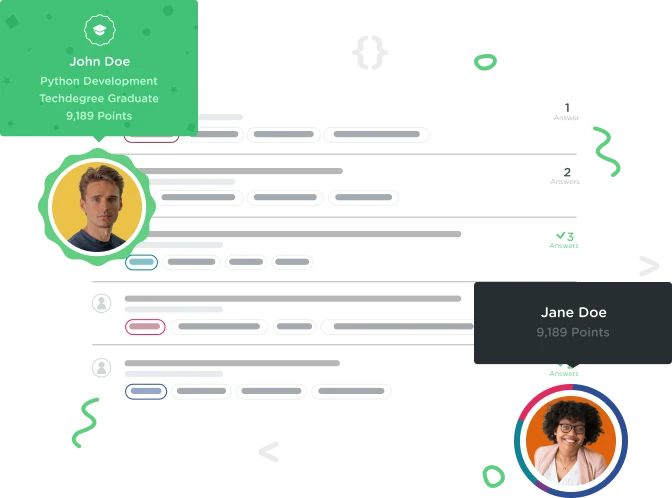
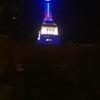
ei2
2,618 PointsHow to use 'echo' to display elements of a multidimensional array.
How do you use echo
to display multiple elements of a multidimensional array?
What is the syntax? For example:
echo $contacts[0]['name'];
Will display the name
of element 0
.
But,
echo $contacts[0]['name']['email'];
Will not display name
and email
....what is going on?
<?php
//edit this array
$contacts = array(
(array ('name' => 'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com')),
(array ('name' => 'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com')),
(array ('name' => 'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com')),
(array ('name' => 'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com')),
);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo $contacts[0]['name'] ['email'];
echo $contacts[1]['name'] ['email'];
echo $contacts[2]['name'] ['email'];
echo $contacts[3]['name'] ['email'];
echo "</ul>\n";
4 Answers

Tylor Boisvert
12,421 PointsWhat are you getting in your code preview? It looks like you would be getting the right output but because of the nature of the challenge, the raw text output doesn't count. Try surrounding your output in <li> tags using concatenation. I was able to pass the challenge with this code. The array is the same two dimensional array I just used square brackets.
<?php
$contacts = array(['name' =>'Alena Holligan', 'email' =>'alena.holligan@teamtreehouse.com'],
['name' =>'Dave McFarland', 'email' =>'dave.mcfarland@teamtreehouse.com'],
['name' =>'Treasure Porth', 'email' =>'treasure.porth@teamtreehouse.com'],
['name' =>'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com']);
echo "<ul>\n";
echo "<li>" . $contacts[0]['name'] . " : " . $contacts[0]['email'] . "</li>\n";
echo "<li>" . $contacts[1]['name'] . " : " . $contacts[1]['email'] . "</li>\n";
echo "<li>" . $contacts[2]['name'] . " : " . $contacts[2]['email'] . "</li>\n";
echo "<li>" . $contacts[3]['name'] . " : " . $contacts[3]['email'] . "</li>\n";
echo "</ul>\n";`

corbinb
2,655 PointsThis worked for me. I didn't realize that I needed to concatenate at the beginning and end of each echo.

KRIS NIKOLAISEN
54,972 PointsYou will have to specify each value individually ($contacts[0]['name']
, $contacts[0]['email']
) and use concatenation.
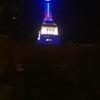
ei2
2,618 PointsOk...when I use concatenation still is not working...getting a result of "Bummer: Try Again!" lol
<?php
//edit this array
$contacts = array(
array('name'=>'Alena Holligan', 'email' => 'alena.holligan@teamtreehouse.com'),
array('name'=>'Dave McFarland', 'email' => 'dave.mcfarland@teamtreehouse.com'),
array('name'=>'Treasure Porth', 'email' => 'treasure.porth@teamtreehouse.com'),
array('name'=>'Andrew Chalkley', 'email' => 'andrew.chalkley@teamtreehouse.com'),
);
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo $contacts[0]['name'] . $contacts[0]['email'];
echo $contacts[1]['name'] . $contacts[1]['email'];
echo $contacts[2]['name'] . $contacts[2]['email'];
echo $contacts[3]['name'] . $contacts[3]['email'];
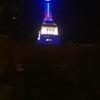
ei2
2,618 PointsOh, ok. Yes, that does seem to work. The fact that you have to keep the <li> elements, does seem to help out. Thanks!

Tylor Boisvert
12,421 PointsRight on glad it helped, you're welcome.
Tylor Boisvert
12,421 PointsTylor Boisvert
12,421 PointsYou would use either 'name' or 'email' as the key after the first index value and concatenate.
When you do this...
...you are looking for the 'email' key as if it were a three dimensional array and returning a single value. Hope this helps.