Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial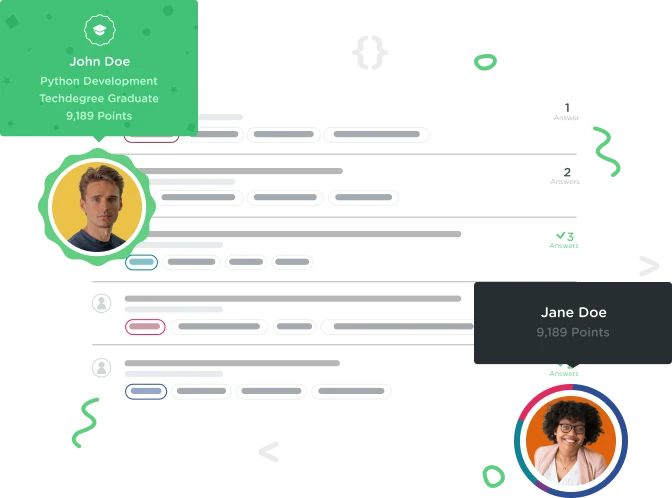
Fred Lawton
5,904 PointsHow to use from and import in Python3?
I'm confused on using just import vs. using from and import.
For example: from sklearn import datasets
should import datasets from the sklearn module. Also, now when I use datasets, I just call datasets
.
However, if I use import sklearn
then I must call sklearn.datasets
to use datasets.
In this case I don't think datasets is a function, I think it's a module that also has a function load_diabetes
.
If I use from sklearn import datasets
followed by '''diabetes = datasets.load_diabetes()
`` the code works.
If I use import sklearn
followed by diabetes = sklearn.datasets.load_diabetes()
I get an error undefined variable from import: datasets.
What am I not understanding?
1 Answer
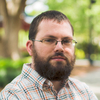
Kenneth Love
Treehouse Guest TeacherYeah, datasets
is probably a module inside sklearn
. I'm wondering about your last statement, though, sklearn.diabetes.load_diabetes()
. Shouldn't that middle diabetes
be datasets
?
Fred Lawton
5,904 PointsFred Lawton
5,904 PointsRe '''sklearn.diabetes.load_diabetes()''' you're correct, and I edited the original post.
To clarify, say I have a module (e.g. sklearn) that has modules (e.g. datasets with a function load_diabetes) and functions (e.g. someFunction). If I want to use the functions inside the datasets module, I can either do 1
or 2
or 3
Is the above correct?
Is there a good explanation somewhere how this namespace stuff works out?
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherI don't think #2 is possible and #3 is somewhat redundant.
Any module can, and usually is, a namespace. Say we create a file name
math.py
that has two methods,add()
andmultiply()
.I can import all of math with
import math
Or I can import just the function(s) I want with
from math import add
orfrom math import add, multiply
or, most cleanlySome will argue that the last example here is redundant but it's definitely easiest if you find yourself suddenly not needing one of those functions. You can also do
from math import *
but that potentially clobbers things you have in your current namespace (because your file is a namespace too!) so it's not recommended.OK, so that covers single files.
scikit-learn
is obviously not a single file.You can also create a module from a directory, say
math/
, but you have to have a file in there named__init__.py
. This file is generally blank.Assume the following layout:
Now I can do
from math.add import add
orfrom math.multiply import multiply
. Unless I have some "special" instructions in the__init__.py
, I can't doimport math
and immediately have access to theadd.add()
function. I could dofrom math.add import add
and then I'd haveadd()
as a function directly.Basically, namespaces are representations of your file system, plus two special ones
locals()
andglobals()
that exist while you're running scripts or in the interpreter. It can be a little confusing but it generally starts to sink in pretty quickly once you start messing with it.