Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial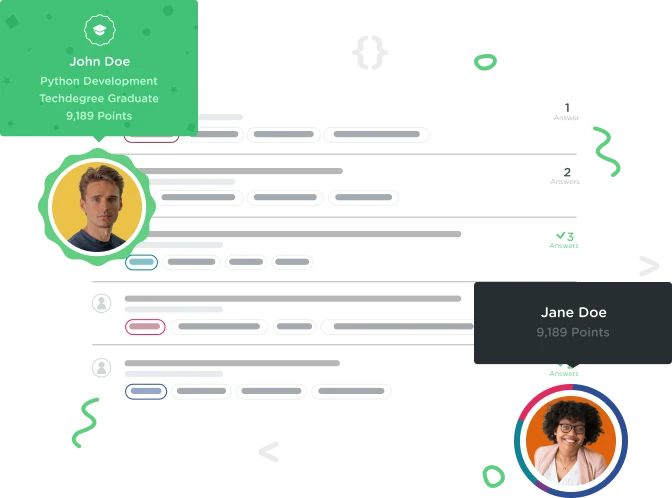

kemishobowale
3,627 PointsHow to use the contains method and toLowerCase method together
The toLowerCase method doesn't appear to be working and I can't figure out why? What am I doing wrong? Entering Pig gets through when i do not want it to.
import java.io.Console;
public class TreeStory {
public static void main(String[] args) {
Console console = System.console();
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here:
*/
String ageAsString = console.readLine("How old are you? ");
int age = Integer.parseInt(ageAsString);
if (age < 13) {
//Insert exit code
console.printf("Sorry you must be at least 13 to use this program.\n");
System.exit(0);
}
String name = console.readLine("Tell me your name: ");
String adjective = console.readLine("Enter an adjective: ");
String noun;
String badWords = "nerd pig jerk";
boolean isInvalidWord;
do {
noun = console.readLine("Enter a noun: ");
isInvalidWord = (noun.toLowerCase().contains(badWords));
if (isInvalidWord) {
console.printf("That language is not allowed. Try again. \n\n");
}
} while(isInvalidWord);
String adverb = console.readLine("Enter an adverb?: ");
String verb = console.readLine("Enter a verb: ");
console.printf("%s is %s %s %s %s\n", name, adjective, noun, adverb, verb);
}
}
2 Answers

Simon Coates
28,694 Pointsit's looking for "nerd pig jerk", not "nerd", "pig", or "jerk" individually. The following demos testing for a series of bad words, while placing the test into a method of its own. The relevant part is the containsBad method.
import java.util.*;
class Main {
public static void main(String[] args) {
Main m = new Main();
m.run();
}
private void run(){
String testIn = "horse apple meow moRoN misanthrope dolphins";
if(containsBad(testIn)){
System.out.println("found a bad word");
} else {
System.out.println("did not find a bad word");
}
}
private boolean containsBad(String testIn){
List<String> badWords = new ArrayList<>(); //should ideally be static
badWords.add("jerk");
badWords.add("moron");
for(String badWord: badWords){
if(testIn.toLowerCase().contains(badWord)){
return true;
}
}
return false;
}
}

kemishobowale
3,627 PointsThanks for your response. My current code can find pig in isolation. The trouble is it can't find Pig with a capital P. The teacher hasn't delved into arrays yet so i would really like to understand this before adding arrays to my code.
Thanks

Simon Coates
28,694 Pointsyour test on validity is okay provided you pass in the correct value to look for (eg. 'pig' and not "nerd pig jerk"). I copied your condition for the following.
class Main {
public static void main(String[] args) {
String noun = "Pig";
System.out.println(noun.toLowerCase().contains("pig"));
noun = "pig";
System.out.println(noun.toLowerCase().contains("pig"));
noun = "any string containing pIG.";
System.out.println(noun.toLowerCase().contains("pig"));
noun = "doesn't contain";
System.out.println(noun.toLowerCase().contains("pig"));
}
}
produces:
true
true
true
false
if you don't want to use arrays, you could use ||. Eg. something like
String lowerNoun = noun.toLowerCase();
boolean isValid = lowerNoun.contains("pig") ||
lowerNoun.contains("dolt") ||
lowerNoun.contains("imbecile");