Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial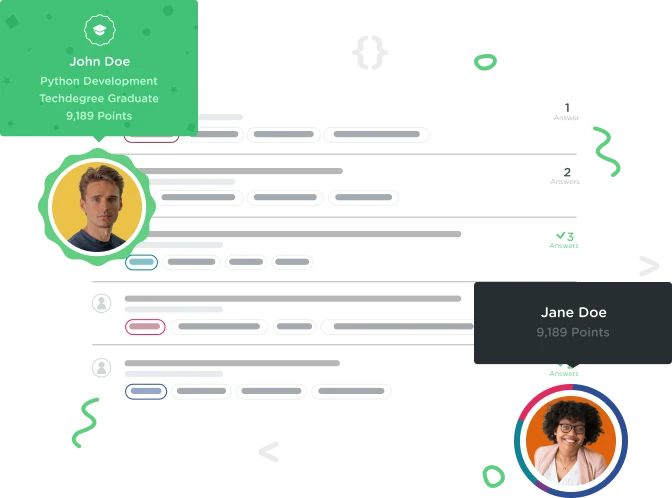

Ashish Mittal
2,302 Pointshow to use the google map matrix api for calculating the distance between two points in java... a big treat is promise
a big treat is promised .its a matter of job.
1 Answer
Christopher Augg
21,223 PointsAshish,
A good place to start is to look at the Documentation for Google Distance Matrix API.
You will need an API Server key. Follow the documentation provided and it will help you get what is needed.
Please complete the weather app for Android or IOS if you have not completed them as they help you learn one way to use an API with OKHTTP and forecast.io. There are many other ways with many different APIs out there. You can google around if you do not like these.
An issue you might run into is an issue with the url to uri encoding. You can look over this reference : URI Encode
A quick fix for the JSON url request is to change the pipe characters | to %7c like the following:
"https://maps.googleapis.com/maps/api/distancematrix/json?origins=Vancouver+BC%7CSeattle&destinations=San+Francisco%7CVictoria+BC&mode=bicycling&language=fr-FR&key=" + API_KEY;
I will give you an example using just java within Eclipse. However, you will need to get your own API key. You will also need to get the two jar files required to add to your build path. Of course, when you decide to do this within Android Studio, use the same method as provided in the weather app by adding the OKhttp dependencies within gradle. And similar coding that was provided within that project.
Download the following files from :
Then add them to your project within Eclipse:
- Create a folder called lib in your project folder.
- Copy to this folder all the jar files you need.
- Refresh your project in eclipse.
- Select all the jar files, then right click on one of them and select Build Path -> Add to Build Path.
Now create the following class in your project and add your own API key:
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import java.io.IOException;
public class GoogMatrixRequest {
private static final String API_KEY = "need your key here";
OkHttpClient client = new OkHttpClient();
public String run(String url) throws IOException {
Request request = new Request.Builder()
.url(url)
.build();
Response response = client.newCall(request).execute();
return response.body().string();
}
public static void main(String[] args) throws IOException {
GoogMatrixRequest request = new GoogMatrixRequest();
String url_request = "https://maps.googleapis.com/maps/api/distancematrix/json?origins=Vancouver+BC%7CSeattle&destinations=San+Francisco%7CVictoria+BC&mode=bicycling&language=fr-FR&key=" + API_KEY;
String response = request.run(url_request);
System.out.println(response);
}
}
This will get you access to the data and print it out to the console like this:
{
"destination_addresses" : [ "San Francisco, Californie, Γtats-Unis", "Victoria, BC, Canada" ],
"origin_addresses" : [ "Vancouver, BC, Canada", "Seattle, Washington, Γtats-Unis" ],
"rows" : [
{
"elements" : [
{
"distance" : {
"text" : "1Β 706 km",
"value" : 1705627
},
"duration" : {
"text" : "3 jours 19 heures",
"value" : 327031
},
"status" : "OK"
},
{
"distance" : {
"text" : "139 km",
"value" : 138549
},
"duration" : {
"text" : "6 heures 42 minutes",
"value" : 24147
},
"status" : "OK"
}
]
},
{
"elements" : [
{
"distance" : {
"text" : "1Β 449 km",
"value" : 1449084
},
"duration" : {
"text" : "3 jours 4 heures",
"value" : 274619
},
"status" : "OK"
},
{
"distance" : {
"text" : "146 km",
"value" : 146496
},
"duration" : {
"text" : "2 heures 52 minutes",
"value" : 10324
},
"status" : "OK"
}
]
}
],
"status" : "OK"
}
Now you should be able to figure out the rest by going back over the documentation and trying some things out. For example:
- You could create some variables for location and create your string to work with many locations.
- You could create a new class to hold data just like we did in the weather app project.
- What kind of views would you use to present this data if you were using android?
Hope this helps.
Regards,
Chris