Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial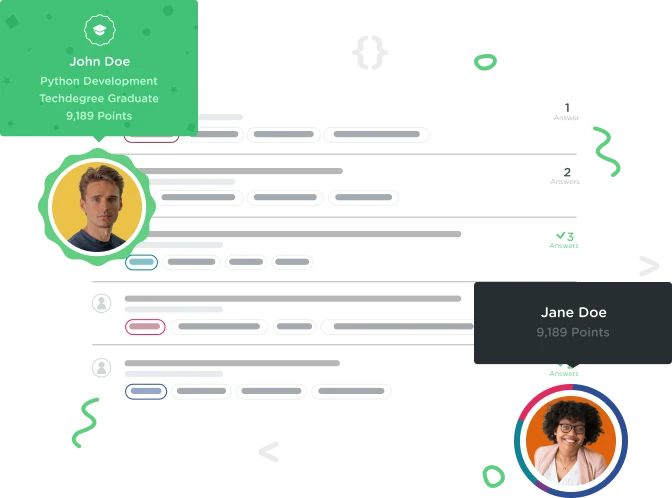
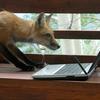
Daniel McNeil
Full Stack JavaScript Techdegree Graduate 26,471 PointsHow to use validation service in Techdegree project: build-rest-api-with-express-v1
I am trying to use the validation service in the angular front end application for the Techdegree project "Build a Course Rating API with Express" to post validation (and login) errors to the screen. According to the project instructions:
Validation errors are returned in the expected format.
{ "message": "Validation Failed", "errors": { "property": [ { "code": "", "message": "" }, ... ] } }
I created a route that returns a JSON object in the above format if someone enters a invalid username (mid.authRequired returns res.currentUser as an error message or the currently authenticated user):
users.get('/', mid.authRequired, (req, res, next) => {
if (res.currentUser === 'invalid username') {
res.status = 400;
let error = { "message": "Validation Failed", "errors": { "property": [ { "code": "login_failure", "message": "Invalid User Name" } ] } };
res.json(error);
} else if (res.currentUser === 'invalid password') {
res.json({data: ['Invalid Password']});
} else {
Users.find({emailAddress: res.currentUser}).exec((error, user) => {
if (error) {
return next(error);
} else {
res.status = 200;
res.json({data: user});
}
});
}
});
When I use Postman to simulate a bad username I get back this JSON object:
{
"message": "Validation Failed",
"errors": {
"property": [
{
"code": "login_failure",
"message": "Invalid User Name"
}
]
}
}
When I try to login with a bad username the error message I get is:
Unexpected Error
Message not available. Please see the console for more details.
I get this message because I am not activating the validation service. I did not write (and am not allowed to modify) the angular application (which contains the validation service) that comes with the project. Can someone tell me what I need to do to use the validation service?
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsDaniel,
Do you see where you're reading "property" in the example instructions? That's actually not supposed to be taken so literally, it should be the name of whatever property has the error. So it should look more like:
In that format, the validation service should pick up those errors.